c语言实现判断一个目录下是否存在指定的文件
时间: 2024-09-12 09:17:44 浏览: 19
在C语言中,判断一个目录下是否存在指定的文件,可以使用标准库中的`opendir`和`readdir`函数来遍历目录,然后用`stat`函数来获取文件的属性信息。以下是一个简单的示例代码,演示了如何实现这个功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <dirent.h>
#include <sys/stat.h>
int file_exists(const char *filename) {
struct stat st;
// 检查文件是否存在于指定的路径
if(stat(filename, &st) == 0) {
// 如果stat函数返回0,说明文件存在
return 1;
} else {
// 如果返回非0值,则文件不存在
return 0;
}
}
int main() {
const char *dir_name = "/path/to/directory";
const char *file_name = "example.txt";
// 构造完整的文件路径
char full_path[260];
strcpy(full_path, dir_name);
strcat(full_path, "/");
strcat(full_path, file_name);
// 判断文件是否存在
if (file_exists(full_path)) {
printf("文件存在。\n");
} else {
printf("文件不存在。\n");
}
return 0;
}
```
在上述代码中,`file_exists`函数使用`stat`函数来获取指定路径的文件属性,如果`stat`函数成功返回,说明文件存在。注意,为了能够正确地使用`stat`函数,需要确保传入的文件路径是正确的,并且程序有权限访问该路径。
需要注意的是,如果目录不存在或者程序没有足够的权限访问该目录,`stat`函数可能会失败。因此,在实际使用时需要进行适当的错误处理。
相关推荐
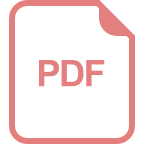
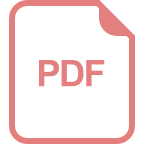
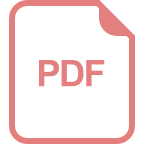














