用VHDL语言描写一个十进制减法计数器
时间: 2024-01-02 11:03:21 浏览: 214
以下是使用VHDL语言描述一个十进制减法计数器的代码示例:
```vhdl
-- 顶层文件
entity top is
port (
clk : in std_logic;
reset : in std_logic;
enable : in std_logic;
count_out : out std_logic_vector(3 downto 0)
);
end top;
architecture Behavioral of top is
signal clk_div : std_logic;
signal count : std_logic_vector(3 downto 0);
begin
-- 分频器
process(clk, reset)
begin
if reset = '1' then
clk_div <= '0';
elsif rising_edge(clk) then
clk_div <= not clk_div;
end if;
end process;
-- 计数器
process(clk_div, reset)
begin
if reset = '1' then
count <= (others => '0');
elsif rising_edge(clk_div) and enable = '1' then
if count = "0000" then
count <= "1001"; -- 初始化为9
else
count <= std_logic_vector(unsigned(count) - 1);
end if;
end if;
end process;
-- 数码管
count_out <= count;
end Behavioral;
-- 分频器
entity divider is
port (
clk : in std_logic;
clk_div : out std_logic
);
end divider;
architecture Behavioral of divider is
signal counter : integer range 0 to 9999 := 0;
begin
process(clk)
begin
if rising_edge(clk) then
if counter = 9999 then
counter <= 0;
clk_div <= not clk_div;
else
counter <= counter + 1;
end if;
end if;
end process;
end Behavioral;
-- 计数器
entity counter is
port (
clk : in std_logic;
reset : in std_logic;
enable : in std_logic;
count_out : out std_logic_vector(3 downto 0)
);
end counter;
architecture Behavioral of counter is
signal count : std_logic_vector(3 downto 0);
begin
process(clk, reset)
begin
if reset = '1' then
count <= (others => '0');
elsif rising_edge(clk) and enable = '1' then
if count = "0000" then
count <= "1001"; -- 初始化为9
else
count <= std_logic_vector(unsigned(count) - 1); end if;
end if;
end process;
count_out <= count;
end Behavioral;
-- 数码管
entity display is
port (
count : in std_logic_vector(3 downto 0);
display_out : out std_logic_vector(6 downto 0)
);
end display;
architecture Behavioral of display is
begin
process(count)
begin
case count is
when "0000" => display_out <= "1000000"; -- 数字0
when "0001" => display_out <= "1111001"; -- 数字1
when "0010" => display_out <= "0100100"; -- 数字2
when "0011" => display_out <= "0110000"; -- 数字3
when "0100" => display_out <= "0011001"; -- 数字4
when "0101" => display_out <= "0010010"; -- 数字5
when "0110" => display_out <= "0000010"; -- 数字6
when "0111" => display_out <= "1111000"; -- 数字7
when "1000" => display_out <= "0000000"; -- 数字8
when "1001" => display_out <= "0010000"; -- 数字9
when others => display_out <= "1111111"; -- 显示空
end case;
end process;
end Behavioral;
```
阅读全文
相关推荐
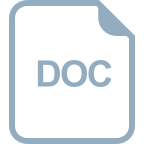
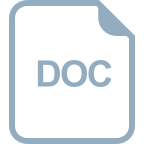
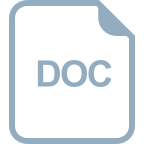

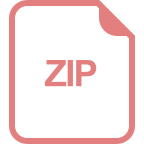
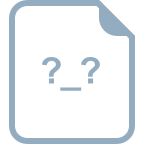
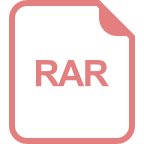
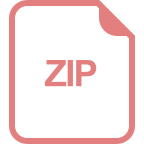

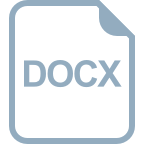
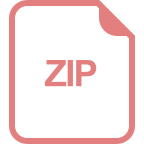
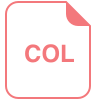


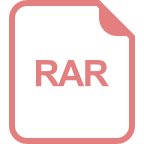
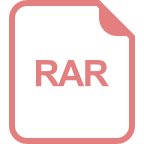
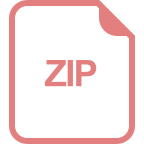
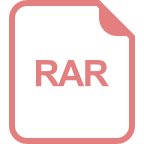