tf.keras.layers.conditional
时间: 2023-10-31 13:59:39 浏览: 117
Conditional layer in TensorFlow's Keras API allows you to conditionally apply different layers based on some input. It is useful when you want to create a model that has different behavior depending on certain conditions.
The `tf.keras.layers.Conditional` layer is not a built-in layer in TensorFlow. However, you can achieve similar functionality using other available layers and custom model construction techniques. One way to implement conditional behavior is by using the `tf.keras.layers.Concatenate` layer along with the `tf.keras.layers.Lambda` layer.
Here's an example of how you can create a conditional layer using these components:
```python
import tensorflow as tf
# Define your conditional inputs
condition = tf.keras.Input(shape=(1,), dtype=tf.bool)
input_1 = tf.keras.Input(shape=(10,))
input_2 = tf.keras.Input(shape=(20,))
# Create a conditional layer
concatenated_inputs = tf.keras.layers.Concatenate(axis=-1)([input_1, input_2])
output = tf.keras.layers.Lambda(lambda x: tf.where(condition, x[:, :10], x[:, 10:]))(concatenated_inputs)
# Create the model
model = tf.keras.Model(inputs=[condition, input_1, input_2], outputs=output)
```
In this example, we have a condition input that determines which part of the concatenated inputs should be selected. The `Lambda` layer uses a lambda function to conditionally select either the first 10 elements or the last 20 elements from the concatenated inputs based on the condition input.
You can customize this example based on your specific requirements and adjust the condition and inputs according to your model's needs.
阅读全文
相关推荐
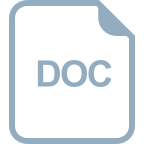
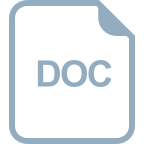
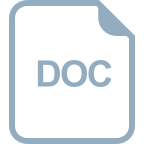
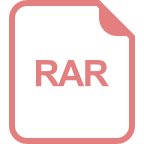
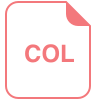
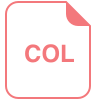
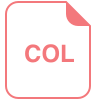


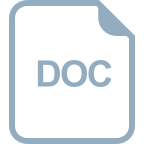
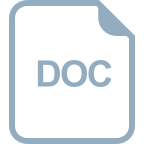
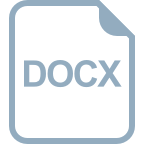
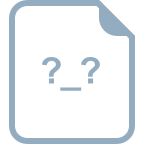
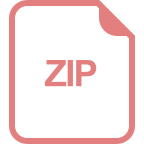