如何使用Python的urllib和BeautifulSoup库抓取网页中的新闻标题和日期,并将这些数据保存到TXT文件中?请提供完整的代码示例。
时间: 2024-11-03 16:09:44 浏览: 67
要实现使用Python的urllib和BeautifulSoup库抓取网页中的新闻标题和日期,并保存到TXT文件中,首先需要了解HTTP请求和HTML解析的基本知识。在这个过程中,urllib库用于发送HTTP请求并接收响应,而BeautifulSoup则用于解析响应内容并提取所需数据。以下是一个详细的代码示例,演示了如何完成这一过程:
参考资源链接:[Python使用urllib和BeautifulSoup抓取网页数据并存入txt](https://wenku.csdn.net/doc/4zxhwoz046?spm=1055.2569.3001.10343)
首先,我们需要导入必要的模块,并定义发送HTTP请求的函数。在这个例子中,我们使用urllib.request模块中的Request和urlopen方法来获取网页数据:
```python
import urllib.request
from bs4 import BeautifulSoup
def get_info(url):
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
request = urllib.request.Request(url, headers=headers)
with urllib.request.urlopen(request) as response:
return response.read()
```
接下来,我们需要解析HTML页面,提取新闻标题和日期。这里使用BeautifulSoup来解析HTML,并通过CSS选择器定位到包含新闻信息的标签:
```python
def parse_html(html):
soup = BeautifulSoup(html, 'lxml')
news_list = soup.find_all('span', class_='column-news-title')
date_list = soup.find_all('span', class_='column-news-date')
news_dict = {title.get_text(strip=True): date.get_text(strip=True) for title, date in zip(news_list, date_list)}
return news_dict
```
最后,我们将抓取到的数据写入到TXT文件中。为了保存方便,我们可以选择将数据转换为JSON格式:
```python
def save_to_txt(news_dict):
with open('xinwen.txt', 'w', encoding='utf-8') as ***
***
```
在主程序中,我们需要遍历网页列表,对每个URL调用上述函数:
```python
if __name__ == '__main__':
urls = ['***{}'.format(i) for i in range(1, 21)]
for url in urls:
html = get_info(url)
news_dict = parse_html(html)
save_to_txt(news_dict)
```
以上代码演示了如何使用urllib发送HTTP请求,BeautifulSoup解析HTML页面,并将提取的数据保存到TXT文件中。需要注意的是,实际使用时,应当遵守目标网站的爬虫政策,避免对网站造成不必要的负担。此外,代码中省略了异常处理逻辑,实际应用时应增加对应的异常处理来提高程序的健壮性。
参考资源链接:[Python使用urllib和BeautifulSoup抓取网页数据并存入txt](https://wenku.csdn.net/doc/4zxhwoz046?spm=1055.2569.3001.10343)
阅读全文
相关推荐
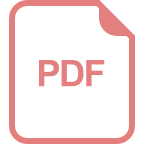
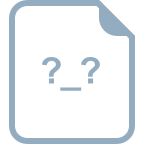
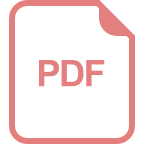














