请描述如何使用Python的urllib库发送HTTP请求并结合BeautifulSoup库解析HTML,以抓取网页中指定CSS类的新闻标题和日期信息,并将这些数据以字典形式保存到TXT文件中。
时间: 2024-11-01 22:09:52 浏览: 39
在进行网页数据抓取时,首先需要使用urllib库发送HTTP请求,获取网页内容。接着,利用BeautifulSoup库解析HTML文档,提取出我们感兴趣的数据。以下是一个具体的示例,展示了如何实现这一过程:
参考资源链接:[Python使用urllib和BeautifulSoup抓取网页数据并存入txt](https://wenku.csdn.net/doc/4zxhwoz046?spm=1055.2569.3001.10343)
1. 导入必要的库:
```python
import urllib.request
from bs4 import BeautifulSoup
import json
```
2. 定义一个函数,该函数使用urllib库发送HTTP请求,并返回网页内容:
```python
def get_page_content(url):
headers = {'User-Agent': 'Mozilla/5.0'}
request = urllib.request.Request(url, headers=headers)
with urllib.request.urlopen(request) as response:
return response.read().decode('utf-8')
```
3. 使用BeautifulSoup解析网页内容,并提取新闻标题和日期:
```python
def parse_news(html):
soup = BeautifulSoup(html, 'lxml')
news_list = []
for item in soup.find_all('span', class_='column-news-title'):
title = item.text
date = item.find_next('span', class_='column-news-date').text
news_list.append({'title': title, 'date': date})
return news_list
```
4. 主程序部分,遍历多个新闻页面,提取数据,并将结果保存到TXT文件:
```python
def main(urls):
results = []
for url in urls:
html = get_page_content(url)
news = parse_news(html)
results.extend(news)
with open('xinwen.txt', 'w', encoding='utf-8') as f:
json.dump(results, f, ensure_ascii=False, indent=4, sort_keys=True)
```
5. 定义URL列表,并调用主函数:
```python
urls = ['***{}'.format(i) for i in range(1, 21)]
main(urls)
```
在上述代码中,我们首先定义了一个获取网页内容的函数`get_page_content`,通过设置请求头来模拟浏览器访问。然后,定义了`parse_news`函数来解析网页内容,提取出新闻标题和日期,并将它们作为字典存储在列表中。最后,我们定义了一个主函数`main`,用于遍历URL列表,提取数据,并将所有新闻信息以JSON格式保存到TXT文件中。
通过这个示例,你可以学习到如何结合使用urllib和BeautifulSoup库来抓取网页中的数据,并将这些数据有效地保存到本地文件。这不仅可以帮助你掌握网络爬虫的基本原理,还能提升你在数据抓取和处理方面的能力。如果你想进一步深入学习,可以参阅《Python使用urllib和BeautifulSoup抓取网页数据并存入txt》这份资料,它包含了更详细的步骤和解释,能够帮助你更好地理解和应用这些技术。
参考资源链接:[Python使用urllib和BeautifulSoup抓取网页数据并存入txt](https://wenku.csdn.net/doc/4zxhwoz046?spm=1055.2569.3001.10343)
阅读全文
相关推荐










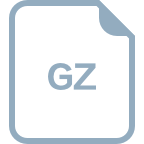







