C语言实现头插法建立一个单链表,并输出
时间: 2024-09-25 21:19:20 浏览: 158
在C语言中,我们可以使用结构体和指针来实现头插法创建单链表。首先,定义一个节点结构体`Node`:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义节点结构体
typedef struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建新节点并插入头部
void insertAtHead(Node** head, int data) {
// 分配内存给新节点
Node* newNode = (Node*)malloc(sizeof(Node));
// 如果链表为空,则新节点既是头节点也是尾节点
if (*head == NULL) {
newNode->data = data;
newNode->next = NULL;
*head = newNode;
} else {
// 新节点的数据放在最前面
newNode->data = data;
newNode->next = *head;
// 更新头节点的指针
*head = newNode;
}
}
// 打印链表
void printList(Node* head) {
while (head != NULL) {
printf("%d -> ", head->data);
head = head->next;
}
printf("NULL\n");
}
int main() {
Node* head = NULL; // 初始化链表头节点
// 插入元素
insertAtHead(&head, 5); // 第一次插入 5
insertAtHead(&head, 3); // 插入 3
insertAtHead(&head, 7); // 插入 7
// 输出链表
printList(head);
return 0;
}
```
在这个示例中,`insertAtHead`函数用于在链表头部添加新节点,`printList`函数用于遍历链表并打印每个节点的数据。在`main`函数中,我们初始化了链表并插入了一些元素。
运行这个程序会得到如下输出(假设输入顺序不变):
```
7 -> 3 -> 5 -> NULL
```
阅读全文
相关推荐
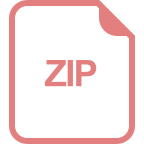
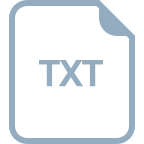
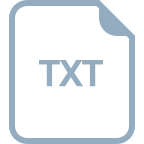















