rs485通讯协议代码详解
时间: 2023-09-02 07:07:16 浏览: 249
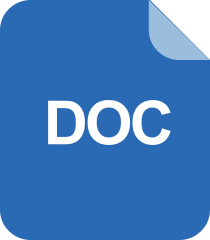
RS485通信协议详解

RS485通讯协议是一种串行通讯协议,常用于工业自动化、智能家居等领域。以下是一个基于C语言的RS485通讯协议代码示例,详解如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
/* 定义串口设备 */
#define SERIAL_DEV "/dev/ttyUSB0"
/* 定义通讯参数 */
#define BAUD_RATE B9600
#define DATA_BITS CS8
#define PARITY_NONE 0
#define STOP_BITS 1
/* 定义RS485控制信号 */
#define RS485_ENABLE 1
#define RS485_DISABLE 0
int serial_fd;
/* 打开串口设备函数 */
int open_serial_device()
{
struct termios options;
serial_fd = open(SERIAL_DEV, O_RDWR | O_NOCTTY | O_NDELAY);
if (serial_fd < 0)
{
perror("open_serial_device(): Unable to open serial device\n");
return -1;
}
fcntl(serial_fd, F_SETFL, 0);
tcgetattr(serial_fd, &options);
cfsetispeed(&options, BAUD_RATE);
cfsetospeed(&options, BAUD_RATE);
options.c_cflag &= ~CSIZE;
options.c_cflag |= DATA_BITS;
options.c_cflag &= ~PARENB;
options.c_iflag &= ~INPCK;
options.c_cflag &= ~CRTSCTS;
options.c_cc[VMIN] = 1;
options.c_cc[VTIME] = 0;
options.c_cflag &= ~CSTOPB;
tcsetattr(serial_fd, TCSANOW, &options);
return 0;
}
/* 设置RS485控制信号函数 */
void set_rs485_control(int enable)
{
int fd;
struct termios termios_options;
fd = open(SERIAL_DEV, O_RDWR | O_NOCTTY);
if (fd < 0)
{
perror("set_rs485_control(): Unable to open serial device\n");
return;
}
tcgetattr(fd, &termios_options);
if (enable)
{
termios_options.c_cflag |= (CLOCAL | CREAD);
termios_options.c_cflag &= ~CSIZE;
termios_options.c_cflag |= CS8;
termios_options.c_cflag &= ~PARENB;
termios_options.c_cflag &= ~CSTOPB;
termios_options.c_cflag &= ~CRTSCTS;
termios_options.c_iflag &= ~(IXON | IXOFF | IXANY);
termios_options.c_iflag &= ~(ICANON | ECHO | ECHOE | ISIG);
termios_options.c_oflag &= ~OPOST;
termios_options.c_cc[VMIN] = 1;
termios_options.c_cc[VTIME] = 0;
ioctl(fd, TIOCMGET, &termios_options.c_cflag);
termios_options.c_cflag |= TIOCM_RTS;
termios_options.c_cflag &= ~TIOCM_DTR;
tcsetattr(fd, TCSANOW, &termios_options);
}
else
{
termios_options.c_cflag &= ~(CLOCAL | CREAD);
termios_options.c_iflag &= ~(IXON | IXOFF | IXANY);
termios_options.c_iflag &= ~(ICANON | ECHO | ECHOE | ISIG);
tcsetattr(fd, TCSANOW, &termios_options);
}
close(fd);
}
/* 发送数据函数 */
int send_data(unsigned char *data, int len)
{
int ret;
set_rs485_control(RS485_ENABLE);
ret = write(serial_fd, data, len);
set_rs485_control(RS485_DISABLE);
return ret;
}
/* 接收数据函数 */
int receive_data(unsigned char *data, int len, int timeout)
{
int ret;
fd_set rfds;
struct timeval tv;
FD_ZERO(&rfds);
FD_SET(serial_fd, &rfds);
tv.tv_sec = timeout / 1000;
tv.tv_usec = (timeout % 1000) * 1000;
set_rs485_control(RS485_ENABLE);
ret = select(serial_fd + 1, &rfds, NULL, NULL, &tv);
if (ret < 0)
{
perror("receive_data(): select() failed\n");
return -1;
}
else if (ret == 0)
{
perror("receive_data(): Timeout occurred\n");
return -1;
}
ret = read(serial_fd, data, len);
set_rs485_control(RS485_DISABLE);
return ret;
}
int main()
{
unsigned char cmd[] = {0x01, 0x03, 0x00, 0x00, 0x00, 0x02, 0xC4, 0x0B};
open_serial_device();
send_data(cmd, sizeof(cmd));
sleep(1);
unsigned char response[1024];
receive_data(response, sizeof(response), 1000);
return 0;
}
```
代码中,`open_serial_device()`函数用于打开串口设备并设置通讯参数;`set_rs485_control()`函数用于设置RS485控制信号,使串口设备处于发送或接收状态;`send_data()`函数用于发送数据;`receive_data()`函数用于接收数据。其中,`send_data()`和`receive_data()`函数均会在发送或接收完成后自动将串口设备设置为接收状态。
以上代码仅供参考,实际使用时还需根据具体情况进行修改。
阅读全文
相关推荐
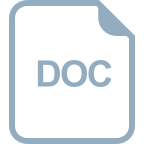



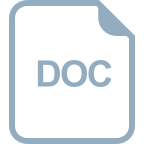
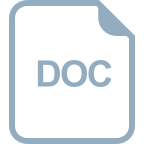
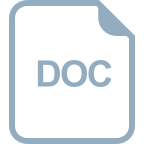
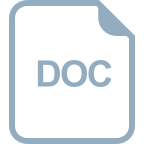
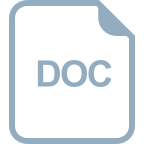
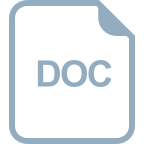
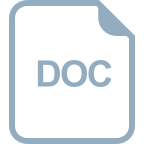
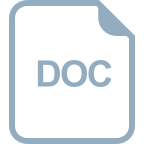
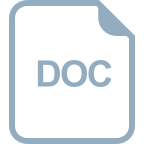
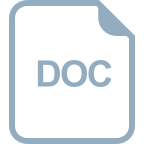
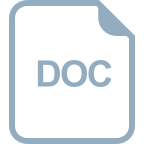
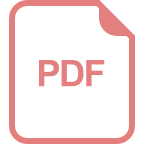