last_segment = path.split("/")[-2]这句话的意思是
时间: 2023-06-24 21:09:17 浏览: 62
这句话的意思是从一个路径字符串中提取出倒数第二个斜杠后面的那一部分作为一个新的字符串。具体来说,它将路径字符串(如"/a/b/c/d/e.txt")按照斜杠"/"进行分割,得到一个字符串列表["", "a", "b", "c", "d", "e.txt"],然后取这个列表的倒数第二个元素"d",作为一个新的字符串返回。所以,last_segment 就是路径中的倒数第二个目录名。
相关问题
if '*' in source_path: source_path = '/'.join(source_path.split('/')[0:-1]) if not source_path.endswith('/'): source_path = source_path + '/' if not error_path.endswith('/'): error_path = error_path + '/' source_file_list = S3_Utils.list_files_by_bucket(source_bucket, source_path, file_type=None) source_file_list = [S3_Utils.decomp_s3_url(file_arn, segment="KEY") for file_arn in source_file_list] self.s3_move_file(source_bucket, source_file_list, error_bucket, error_path, self.config.kms_key_id)
这段代码也是 Python 代码,它的作用是将源路径中的所有文件移动到错误路径中,并且通过 S3_Utils 工具类中的 list_files_by_bucket 方法获取到源路径中所有的文件列表。如果源路径中存在通配符 `*`,则代码会将通配符前面的部分作为实际的路径,并将通配符去除。如果源路径或错误路径没有以 `/` 结尾,则代码会在路径末尾添加 `/`。最后,代码调用 s3_move_file 方法将源路径中的文件移动到错误路径中,并且使用 config 中指定的 KMS Key ID 进行加密。具体的实现细节需要查看 s3_move_file 和 list_files_by_bucket 方法的源代码。
import tensorflow as tf import tensorflow_hub as hub from tensorflow.keras import layers import bert import numpy as np from transformers import BertTokenizer, BertModel # 设置BERT模型的路径和参数 bert_path = "E:\\AAA\\523\\BERT-pytorch-master\\bert1.ckpt" max_seq_length = 128 train_batch_size = 32 learning_rate = 2e-5 num_train_epochs = 3 # 加载BERT模型 def create_model(): input_word_ids = tf.keras.layers.Input(shape=(max_seq_length,), dtype=tf.int32, name="input_word_ids") input_mask = tf.keras.layers.Input(shape=(max_seq_length,), dtype=tf.int32, name="input_mask") segment_ids = tf.keras.layers.Input(shape=(max_seq_length,), dtype=tf.int32, name="segment_ids") bert_layer = hub.KerasLayer(bert_path, trainable=True) pooled_output, sequence_output = bert_layer([input_word_ids, input_mask, segment_ids]) output = layers.Dense(1, activation='sigmoid')(pooled_output) model = tf.keras.models.Model(inputs=[input_word_ids, input_mask, segment_ids], outputs=output) return model # 准备数据 def create_input_data(sentences, labels): tokenizer = bert.tokenization.FullTokenizer(vocab_file=bert_path + "trainer/vocab.small", do_lower_case=True) # tokenizer = BertTokenizer.from_pretrained('bert-base-uncased') input_ids = [] input_masks = [] segment_ids = [] for sentence in sentences: tokens = tokenizer.tokenize(sentence) tokens = ["[CLS]"] + tokens + ["[SEP]"] input_id = tokenizer.convert_tokens_to_ids(tokens) input_mask = [1] * len(input_id) segment_id = [0] * len(input_id) padding_length = max_seq_length - len(input_id) input_id += [0] * padding_length input_mask += [0] * padding_length segment_id += [0] * padding_length input_ids.append(input_id) input_masks.append(input_mask) segment_ids.append(segment_id) return np.array(input_ids), np.array(input_masks), np.array(segment_ids), np.array(labels) # 加载训练数据 train_sentences = ["Example sentence 1", "Example sentence 2", ...] train_labels = [0, 1, ...] train_input_ids, train_input_masks, train_segment_ids, train_labels = create_input_data(train_sentences, train_labels) # 构建模型 model = create_model() model.compile(optimizer=tf.keras.optimizers.Adam(lr=learning_rate), loss='binary_crossentropy', metrics=['accuracy']) # 开始微调 model.fit([train_input_ids, train_input_masks, train_segment_ids], train_labels, batch_size=train_batch_size, epochs=num_train_epochs)这段代码有什么问题吗?
这段代码存在一些问题:
1. `bert_path` 的值不应该是 ".ckpt" 结尾的文件路径,而是BERT模型的目录路径,例如:`bert_path = "E:\\AAA\\523\\BERT-pytorch-master\\"`。
2. `vocab_file` 参数应该是BERT模型目录下的 "vocab.txt" 文件路径,而不是训练器目录下的 "vocab.small" 文件路径。例如:`tokenizer = bert.tokenization.FullTokenizer(vocab_file=bert_path + "vocab.txt", do_lower_case=True)`。
3. `create_input_data` 函数中的 `tokenizer` 对象应该是从 `BertTokenizer` 类中导入的。因此,需要将 `from transformers import BertTokenizer` 加入代码开头,并使用 `BertTokenizer.from_pretrained()` 函数来加载BERT的tokenizer。
4. `train_sentences` 和 `train_labels` 应该是训练数据集,但是在代码中缺失了这些数据的定义和加载。
5. `create_input_data` 函数返回的 `labels` 应该是一维的,而不是二维的。因此,需要修改函数 `return` 语句为:`return np.array(input_ids), np.array(input_masks), np.array(segment_ids), np.array(labels).reshape(-1)`。
6. 在 `model.fit` 中,需要指定验证集的参数 `validation_data`,以便在训练过程中评估模型的性能。
综上所述,以下是修改后的代码示例:
```
import tensorflow as tf
import tensorflow_hub as hub
from tensorflow.keras import layers
import bert
import numpy as np
from transformers import BertTokenizer, BertModel
# 设置BERT模型的路径和参数
bert_path = "E:\\AAA\\523\\BERT-pytorch-master\\"
max_seq_length = 128
train_batch_size = 32
learning_rate = 2e-5
num_train_epochs = 3
# 加载BERT模型
def create_model():
input_word_ids = tf.keras.layers.Input(shape=(max_seq_length,), dtype=tf.int32, name="input_word_ids")
input_mask = tf.keras.layers.Input(shape=(max_seq_length,), dtype=tf.int32, name="input_mask")
segment_ids = tf.keras.layers.Input(shape=(max_seq_length,), dtype=tf.int32, name="segment_ids")
bert_layer = hub.KerasLayer(bert_path, trainable=True)
pooled_output, sequence_output = bert_layer([input_word_ids, input_mask, segment_ids])
output = layers.Dense(1, activation='sigmoid')(pooled_output)
model = tf.keras.models.Model(inputs=[input_word_ids, input_mask, segment_ids], outputs=output)
return model
# 准备数据
def create_input_data(sentences, labels):
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
input_ids = []
input_masks = []
segment_ids = []
for sentence in sentences:
tokens = tokenizer.tokenize(sentence)
tokens = ["[CLS]"] + tokens + ["[SEP]"]
input_id = tokenizer.convert_tokens_to_ids(tokens)
input_mask = [1] * len(input_id)
segment_id = [0] * len(input_id)
padding_length = max_seq_length - len(input_id)
input_id += [0] * padding_length
input_mask += [0] * padding_length
segment_id += [0] * padding_length
input_ids.append(input_id)
input_masks.append(input_mask)
segment_ids.append(segment_id)
return np.array(input_ids), np.array(input_masks), np.array(segment_ids), np.array(labels).reshape(-1)
# 加载训练数据
train_sentences = ["Example sentence 1", "Example sentence 2", ...]
train_labels = [0, 1, ...]
train_input_ids, train_input_masks, train_segment_ids, train_labels = create_input_data(train_sentences, train_labels)
# 构建模型
model = create_model()
model.compile(optimizer=tf.keras.optimizers.Adam(lr=learning_rate),
loss='binary_crossentropy',
metrics=['accuracy'])
# 开始微调
model.fit([train_input_ids, train_input_masks, train_segment_ids], train_labels,
batch_size=train_batch_size,
epochs=num_train_epochs,
validation_data=([val_input_ids, val_input_masks, val_segment_ids], val_labels))
```
相关推荐
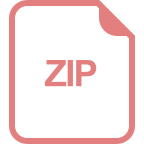
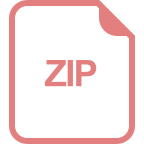













