TypeError: '<' not supported between instances of 'ListNode' and 'ListNode' heappush(self.queue, item) Line 236 in _put (/usr/lib/python3.10/queue.py) self._put(item) Line 150 in put (/usr/lib/python3.10/queue.py) q.put((l.val,l)) Line 28 in m
时间: 2024-03-14 16:47:43 浏览: 17
这个错误是因为你在使用 Python 的 queue 模块时,尝试将一个 ListNode 实例与另一个 ListNode 实例进行比较。Python 中的堆(heap)是一个二叉树的数据结构,并且它要求堆中的元素必须是可比较的。
为了解决这个问题,你需要为 ListNode 类实现一个 __lt__ 方法(即小于号运算符的重载),以便 Python 知道如何比较两个 ListNode 实例。例如:
```
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def __lt__(self, other):
return self.val < other.val
```
在这个例子中,我们定义了一个 __lt__ 方法,使得当比较两个 ListNode 实例时,Python 会比较它们的 val 属性。
你也可以通过将元组作为优先级队列的元素来规避这个问题,例如:
```
q.put((l.val, l))
```
这里我们将 ListNode 实例放在元组的第二个位置,而将 val 属性放在第一个位置,这样就可以避免直接比较 ListNode 实例了。
相关问题
TypeError: '<' not supported between instances of 'numpy.str_' and 'float'
这个错误通常表示你正在尝试使用 `<` 操作符比较一个字符串和一个浮点数,但这些类型不能直接比较。可能是你在某个地方出现了类型不匹配的问题。
你需要检查一下代码,看看哪个变量是字符串类型,哪个变量是浮点数类型,然后确保它们进行比较之前都是同一类型。你可以尝试使用 `float()` 函数将字符串转换为浮点数,或者使用 `str()` 函数将浮点数转换为字符串,以便它们可以进行比较。
E TypeError: '<=' not supported between instances of 'str' and 'datetime.datetime'
这个报错是因为你在比较一个字符串和一个 datetime 对象,而这两者之间是不能直接比较的。你需要将字符串转换成 datetime 对象,然后再进行比较。例如,如果你有一个字符串表示的时间,你可以使用 datetime.strptime() 方法将其转换成 datetime 对象,然后再进行比较。示例如下:
```python
from datetime import datetime
# 将字符串转换成 datetime 对象
time_str = '2023-05-01'
time_obj = datetime.strptime(time_str, '%Y-%m-%d')
# 比较时间
if time_obj <= datetime.now():
print('时间已经过去了')
else:
print('时间还没有到')
```
在上面的示例中,我们先将字符串 '2023-05-01' 转换成 datetime 对象 time_obj,然后再将其和当前时间进行比较。注意,在使用 datetime.strptime() 方法转换时间字符串时,需要指定时间字符串的格式,以便正确地解析时间字符串。
相关推荐
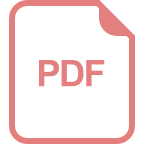
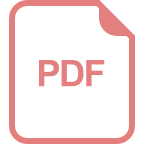
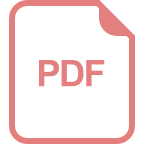












