读取文件夹里的json文件并判断是不是最后一个文件
时间: 2023-12-19 14:04:51 浏览: 29
读取文件夹里的json文件可以使用readdir函数遍历目录,并使用strstr函数判断文件名中是否包含".json"这个字符串,如果包含则说明这个文件是json文件。
下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <dirent.h>
#include <string.h>
int main()
{
DIR *dir;
struct dirent *entry;
int count = 0, json_count = 0;
char *folder_path = "./folder"; // 文件夹路径
dir = opendir(folder_path);
if (dir == NULL) {
perror("opendir");
return EXIT_FAILURE;
}
while ((entry = readdir(dir)) != NULL) {
if (entry->d_type == DT_REG) { // 判断是普通文件
count++;
if (strstr(entry->d_name, ".json") != NULL) { // 判断文件名中是否包含".json"
json_count++;
// 如果需要读取json文件内容,可以在这里打开文件并读取
}
}
}
closedir(dir);
if (json_count > 0) {
printf("There are %d json files in the folder.\n", json_count);
} else {
printf("There is no json file in the folder.\n");
}
if (json_count == count) {
printf("This is the last file.\n");
} else {
printf("This is not the last file.\n");
}
return EXIT_SUCCESS;
}
```
上述代码会统计文件夹中所有的json文件数量和普通文件数量,并判断当前文件是否为最后一个文件。需要注意的是,这种判断方式只适用于文件夹中没有子文件夹的情况。如果需要处理含有子文件夹的情况,可以使用递归的方式遍历整个目录树。
相关推荐
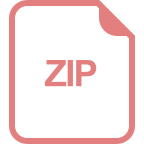
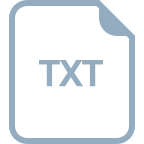
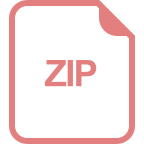














