#include <stdio.h> int main() { double x, y; scanf ("%lf", &x); printf ("f(%.2f) = %.2f\n", x, y); return 0; } { double result; if (x <= 15){ result = 4 * x / 3; } else{ result = 2.5 * x - 10.5; } }
时间: 2023-11-13 16:03:20 浏览: 576
这段代码有一些问题,我来指出并修正:
1. `y` 没有被赋值,应该在计算 `result` 后将其赋值给 `y`。
2. `result` 的声明放在了条件语句外面,应该放在条件语句里面。
3. `main` 函数的开头没有写明返回类型,应该写为 `int main(void)` 或者 `int main()`。
修正后的代码如下:
```c
#include <stdio.h>
int main() {
double x, y, result;
scanf("%lf", &x);
if (x <= 15) {
result = 4 * x / 3;
} else {
result = 2.5 * x - 10.5;
}
y = result;
printf("f(%.2f) = %.2f\n", x, y);
return 0;
}
```
修正后的代码可以正确地计算函数值并输出结果。
相关问题
求两点间的距离#include <stdio.h> #include <math.h> typedef struct { double x, y; } POINT; int main() { POINT a, b; double s, p, q; scanf("%lf %lf", &a.x, &a.y); scanf("%lf %lf", &b.x, &b.y); printf("%g\n", s);
你可以使用勾股定理求解两点之间的距离,具体代码如下:
```c
#include <stdio.h>
#include <math.h>
typedef struct {
double x, y;
} POINT;
int main() {
POINT a, b;
double s, p, q;
scanf("%lf %lf", &a.x, &a.y);
scanf("%lf %lf", &b.x, &b.y);
p = a.x - b.x;
q = a.y - b.y;
s = sqrt(p * p + q * q);
printf("%g\n", s);
return 0;
}
```
其中,p和q分别代表两个点在x轴和y轴上的坐标差值,s为两点之间的距离,使用了数学库中的sqrt函数来求解根号。
#include <stdio.h> #include <math.h> typedef struct { double x, y; } POINT; int main() { POINT a, b; double s, p, q; scanf("%lf %lf", &a.x, &a.y); scanf("%lf %lf", &b.x, &b.y); /* 你提交的代码将被嵌在这里 */ printf("%g\n", s); return 0; }
下面是计算两点间距离并输出的代码:
```c
#include <stdio.h>
#include <math.h>
typedef struct {
double x, y;
} POINT;
int main() {
POINT a, b;
double s, p, q;
scanf("%lf %lf", &a.x, &a.y);
scanf("%lf %lf", &b.x, &b.y);
// 计算两点间距离
s = sqrt(pow(a.x - b.x, 2) + pow(a.y - b.y, 2));
// 输出结果
printf("%g\n", s);
return 0;
}
```
输入格式为两行,每行两个实数,分别代表两个点的坐标。输出格式为一个实数,代表两点间距离。
阅读全文
相关推荐
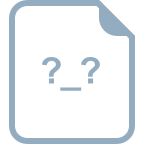














