#include <stdio.h> #include <math.h> #include <stdlib.h> /********** Begin *********/ int main(){ double v; int t; scanf("%lf%d",&v,&t); double x=v*t; double h=9.8*t*t/2; double s=sqrt(x*x+h*h); printf("%.2lf\n",s); return 0; } /********** End *********/
时间: 2023-07-01 11:15:37 浏览: 47
这是一个计算抛体运动轨迹长度的 C 语言程序。它的输入是初速度 v 和时间 t,输出是轨迹长度 s。
程序的实现方法是:先根据初速度和时间计算出水平方向上的位移 x 和竖直方向上的位移 h,然后根据勾股定理求出轨迹长度 s。
程序的主要代码如下:
```c
double x=v*t; // 计算水平方向位移
double h=9.8*t*t/2; // 计算竖直方向位移
double s=sqrt(x*x+h*h); // 计算轨迹长度
printf("%.2lf\n",s); // 输出轨迹长度
```
其中,sqrt 函数是 C 语言中的开方函数,可以通过 #include <math.h> 头文件引入。函数的返回值是一个 double 类型的浮点数,表示输入参数的平方根。
程序的完整代码如下:
相关问题
#include <stdio.h> #include <stdlib.h> #include <math.h>
这段代码是一个C语言的程序头文件,包含了一些常用的函数库,比如stdio.h、stdlib.h和math.h。其中:
- stdio.h包含了标准输入输出函数,比如printf和scanf。
- stdlib.h包含了一些通用的函数,比如malloc、free和exit。
- math.h包含了一些数学函数,比如sin、cos和sqrt等。
#include <stdio.h> #include <math.h> #include <stdlib.h>
这是一个 C 语言的程序的头文件部分,其中包括了常用的标准输入输出库函数和数学库函数。其中:
- stdio.h 是标准输入输出库头文件,包含了 printf、scanf 等函数;
- math.h 是数学库头文件,包含了数学函数,例如 sin、cos、sqrt 等函数;
- stdlib.h 是标准库的头文件,包含了一些通用的函数,例如 malloc、free、exit 等函数。
相关推荐
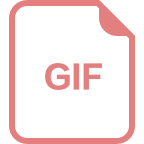
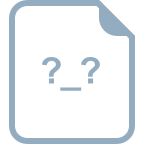
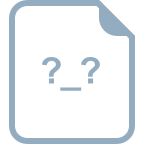













