用c++写一遍上述代码,能直接运行
时间: 2024-01-28 11:02:45 浏览: 56
好的,下面是使用 C++ 实现的代码:
```c++
#include <iostream>
#include <vector>
#include <set>
#include <queue>
using namespace std;
int bfs(int start_x, int start_y, int end_x, int end_y) {
queue<vector<int>> q;
q.push({start_x, start_y, 0}); // 将起始位置加入队列
set<pair<int, int>> visited; // 记录已经搜索过的位置
visited.insert({start_x, start_y});
while (!q.empty()) {
vector<int> cur = q.front();
q.pop();
int x = cur[0], y = cur[1], step = cur[2];
if (x == end_x && y == end_y) // 找到目标位置
return step;
// 尝试所有可能的移动方式,并将能够到达的位置加入队列
for (auto [dx, dy] : vector<vector<int>>{{1, 0}, {-1, 0}, {0, 1}, {0, -1}, {2, 0}, {-2, 0}, {0, 2}, {0, -2}, {2, 2}}) {
int nx = x + dx, ny = y + dy;
if (visited.find({nx, ny}) == visited.end() && 1 <= nx && nx <= end_x + end_y && 1 <= ny && ny <= end_x + end_y) {
visited.insert({nx, ny});
q.push({nx, ny, step + 1});
}
}
}
return -1; // 如果无法到达目标位置,返回-1
}
int backtrack(int x, int y, int end_x, int end_y, set<pair<int, int>>& visited, int step) {
if (x == end_x && y == end_y) // 找到目标位置,返回步数
return step;
int min_step = INT_MAX;
for (auto [dx, dy] : vector<vector<int>>{{1, 0}, {-1, 0}, {0, 1}, {0, -1}, {2, 0}, {-2, 0}, {0, 2}, {0, -2}, {2, 2}}) {
int nx = x + dx, ny = y + dy;
if (visited.find({nx, ny}) == visited.end() && 1 <= nx && nx <= end_x + end_y && 1 <= ny && ny <= end_x + end_y) {
visited.insert({nx, ny});
int cur_step = backtrack(nx, ny, end_x, end_y, visited, step + 1);
if (cur_step != -1)
min_step = min(min_step, cur_step);
visited.erase({nx, ny});
}
}
return min_step == INT_MAX ? -1 : min_step;
}
int min_steps(int start_x, int start_y, int end_x, int end_y) {
set<pair<int, int>> visited;
visited.insert({start_x, start_y});
return backtrack(start_x, start_y, end_x, end_y, visited, 0);
}
int main() {
int start_x = 1, start_y = 1, end_x = 5, end_y = 5;
cout << bfs(start_x, start_y, end_x, end_y) << endl; // 输出最小步数
cout << min_steps(start_x, start_y, end_x, end_y) << endl;
return 0;
}
```
其中,backtrack 函数和之前的 Python 版本一样,使用 visited 集合记录已经访问过的位置。bfs 函数使用了一个队列和一个 visited 集合来实现广度优先搜索,代码结构和之前的 Python 版本也相似。注意,C++ 中使用 set 和 pair 来代替 Python 中的集合和元组。
阅读全文
相关推荐
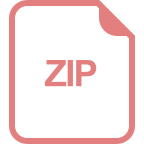
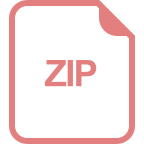
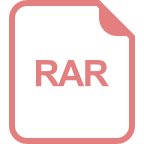
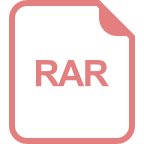
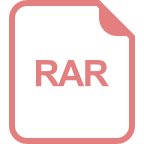
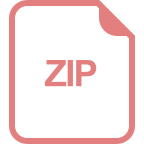
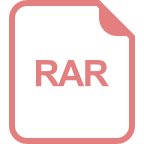
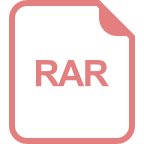
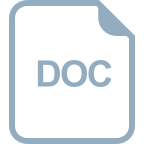
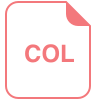
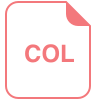
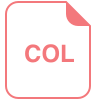
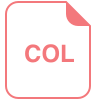
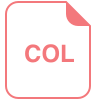

