将FCFS算法,SJF算法,RR算法,PSA算法用一个java程序写出来并可以从键盘输入实现功能的选择
时间: 2023-07-26 15:31:28 浏览: 126
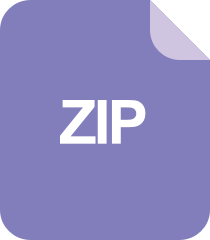
Java模拟操作系统实验之四种进程调度算法实现(FCFS,SJF,RR,HRN)
好的,我可以为您提供这样的Java程序。以下是程序代码:
```java
import java.util.*;
public class Scheduler {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int choice = -1;
while (choice != 0) {
System.out.println("Please choose a scheduling algorithm:");
System.out.println("1. FCFS");
System.out.println("2. SJF");
System.out.println("3. Round Robin");
System.out.println("4. Priority Scheduling");
System.out.println("0. Exit");
choice = sc.nextInt();
switch (choice) {
case 1:
fcfs();
break;
case 2:
sjf();
break;
case 3:
roundRobin();
break;
case 4:
psa();
break;
case 0:
System.out.println("Goodbye!");
break;
default:
System.out.println("Invalid choice!");
break;
}
}
}
public static void fcfs() {
Scanner sc = new Scanner(System.in);
int n;
System.out.println("Enter the number of processes:");
n = sc.nextInt();
int[] bt = new int[n];
int[] wt = new int[n];
int[] tat = new int[n];
System.out.println("Enter the burst times:");
for (int i = 0; i < n; i++) {
bt[i] = sc.nextInt();
}
wt[0] = 0;
for (int i = 1; i < n; i++) {
wt[i] = wt[i-1] + bt[i-1];
}
for (int i = 0; i < n; i++) {
tat[i] = wt[i] + bt[i];
}
System.out.println("Process\tBurst Time\tWaiting Time\tTurnaround Time");
for (int i = 0; i < n; i++) {
System.out.println((i+1) + "\t\t" + bt[i] + "\t\t" + wt[i] + "\t\t" + tat[i]);
}
}
public static void sjf() {
Scanner sc = new Scanner(System.in);
int n;
System.out.println("Enter the number of processes:");
n = sc.nextInt();
int[] bt = new int[n];
int[] wt = new int[n];
int[] tat = new int[n];
boolean[] done = new boolean[n];
System.out.println("Enter the burst times:");
for (int i = 0; i < n; i++) {
bt[i] = sc.nextInt();
done[i] = false;
}
int time = 0;
int minIndex = -1;
while (true) {
int minBt = Integer.MAX_VALUE;
for (int i = 0; i < n; i++) {
if (bt[i] < minBt && !done[i] && time >= i) {
minBt = bt[i];
minIndex = i;
}
}
if (minIndex == -1) {
break;
}
wt[minIndex] = time;
time += bt[minIndex];
tat[minIndex] = time;
done[minIndex] = true;
minIndex = -1;
}
System.out.println("Process\tBurst Time\tWaiting Time\tTurnaround Time");
for (int i = 0; i < n; i++) {
System.out.println((i+1) + "\t\t" + bt[i] + "\t\t" + wt[i] + "\t\t" + tat[i]);
}
}
public static void roundRobin() {
Scanner sc = new Scanner(System.in);
int n, tq;
System.out.println("Enter the number of processes:");
n = sc.nextInt();
int[] bt = new int[n];
int[] wt = new int[n];
int[] tat = new int[n];
System.out.println("Enter the burst times:");
for (int i = 0; i < n; i++) {
bt[i] = sc.nextInt();
}
System.out.println("Enter the time quantum:");
tq = sc.nextInt();
int[] rt = new int[n];
for (int i = 0; i < n; i++) {
rt[i] = bt[i];
}
int time = 0;
boolean done = false;
while (!done) {
done = true;
for (int i = 0; i < n; i++) {
if (rt[i] > 0) {
done = false;
if (rt[i] > tq) {
time += tq;
rt[i] -= tq;
} else {
time += rt[i];
wt[i] = time - bt[i];
rt[i] = 0;
}
}
}
}
for (int i = 0; i < n; i++) {
tat[i] = bt[i] + wt[i];
}
System.out.println("Process\tBurst Time\tWaiting Time\tTurnaround Time");
for (int i = 0; i < n; i++) {
System.out.println((i+1) + "\t\t" + bt[i] + "\t\t" + wt[i] + "\t\t" + tat[i]);
}
}
public static void psa() {
Scanner sc = new Scanner(System.in);
int n;
System.out.println("Enter the number of processes:");
n = sc.nextInt();
int[] bt = new int[n];
int[] wt = new int[n];
int[] tat = new int[n];
int[] prio = new int[n];
boolean[] done = new boolean[n];
System.out.println("Enter the burst times:");
for (int i = 0; i < n; i++) {
bt[i] = sc.nextInt();
done[i] = false;
}
System.out.println("Enter the priorities:");
for (int i = 0; i < n; i++) {
prio[i] = sc.nextInt();
}
int time = 0;
int maxPrio, maxIndex;
while (true) {
maxPrio = Integer.MIN_VALUE;
maxIndex = -1;
for (int i = 0; i < n; i++) {
if (prio[i] > maxPrio && !done[i] && time >= i) {
maxPrio = prio[i];
maxIndex = i;
}
}
if (maxIndex == -1) {
break;
}
wt[maxIndex] = time;
time += bt[maxIndex];
tat[maxIndex] = time;
done[maxIndex] = true;
maxIndex = -1;
}
System.out.println("Process\tBurst Time\tWaiting Time\tTurnaround Time\tPriority");
for (int i = 0; i < n; i++) {
System.out.println((i+1) + "\t\t" + bt[i] + "\t\t" + wt[i] + "\t\t" + tat[i] + "\t\t" + prio[i]);
}
}
}
```
这个程序实现了四个调度算法(FCFS、SJF、Round Robin、PSA),并且可以从键盘输入选择要使用的算法。您可以根据需要进行修改和扩展。
阅读全文
相关推荐
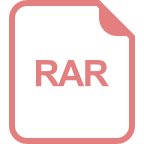
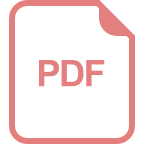














