psa优先调度算法的c语言,FCFS、SJF、PSA、RR、MFS作业调度C语言实现
时间: 2023-07-10 20:08:36 浏览: 114
以下是常见的作业调度算法的C语言实现:
1. FCFS(先来先服务)
```c
#include <stdio.h>
int main()
{
int n, i;
float avg_tat = 0, avg_wt = 0;
int burst[20], tat[20], wt[20];
printf("Enter the number of processes: ");
scanf("%d", &n);
printf("Enter the burst time for each process:\n");
for(i=0; i<n; i++)
{
printf("P[%d]: ", i+1);
scanf("%d", &burst[i]);
}
tat[0] = burst[0];
wt[0] = 0;
for(i=1; i<n; i++)
{
tat[i] = tat[i-1] + burst[i];
wt[i] = tat[i-1];
}
printf("\nProcess\tBurst Time\tTurnaround Time\tWaiting Time\n");
for(i=0; i<n; i++)
{
printf("P[%d]\t%d\t\t%d\t\t%d\n", i+1, burst[i], tat[i], wt[i]);
avg_tat += tat[i];
avg_wt += wt[i];
}
avg_tat /= n;
avg_wt /= n;
printf("\nAverage Turnaround Time: %.2f", avg_tat);
printf("\nAverage Waiting Time: %.2f", avg_wt);
return 0;
}
```
2. SJF(短作业优先)
```c
#include <stdio.h>
#include <stdlib.h>
struct process
{
int id;
int burst;
int arrival;
int tat;
int wt;
};
void swap(struct process *a, struct process *b)
{
struct process temp = *a;
*a = *b;
*b = temp;
}
void sort(struct process arr[], int n)
{
int i, j;
for(i=0; i<n-1; i++)
{
for(j=0; j<n-i-1; j++)
{
if(arr[j].burst > arr[j+1].burst)
{
swap(&arr[j], &arr[j+1]);
}
}
}
}
int main()
{
int n, i, j;
float avg_tat = 0, avg_wt = 0;
struct process p[20];
printf("Enter the number of processes: ");
scanf("%d", &n);
printf("Enter the arrival time and burst time for each process:\n");
for(i=0; i<n; i++)
{
printf("P[%d]: ", i+1);
p[i].id = i+1;
scanf("%d %d", &p[i].arrival, &p[i].burst);
}
sort(p, n);
p[0].tat = p[0].burst;
p[0].wt = 0;
for(i=1; i<n; i++)
{
int sum = 0;
for(j=0; j<i; j++)
{
sum += p[j].burst;
}
p[i].tat = sum + p[i].burst - p[i].arrival;
p[i].wt = p[i-1].wt + p[i-1].burst - p[i].arrival;
if(p[i].wt < 0)
{
p[i].wt = 0;
}
}
printf("\nProcess\tArrival Time\tBurst Time\tTurnaround Time\tWaiting Time\n");
for(i=0; i<n; i++)
{
printf("P[%d]\t%d\t\t%d\t\t%d\t\t%d\n", p[i].id, p[i].arrival, p[i].burst, p[i].tat, p[i].wt);
avg_tat += p[i].tat;
avg_wt += p[i].wt;
}
avg_tat /= n;
avg_wt /= n;
printf("\nAverage Turnaround Time: %.2f", avg_tat);
printf("\nAverage Waiting Time: %.2f", avg_wt);
return 0;
}
```
3. PSA(优先级调度算法)
```c
#include <stdio.h>
#include <stdlib.h>
struct process
{
int id;
int burst;
int priority;
int tat;
int wt;
};
void swap(struct process *a, struct process *b)
{
struct process temp = *a;
*a = *b;
*b = temp;
}
void sort(struct process arr[], int n)
{
int i, j;
for(i=0; i<n-1; i++)
{
for(j=0; j<n-i-1; j++)
{
if(arr[j].priority > arr[j+1].priority)
{
swap(&arr[j], &arr[j+1]);
}
}
}
}
int main()
{
int n, i, j;
float avg_tat = 0, avg_wt = 0;
struct process p[20];
printf("Enter the number of processes: ");
scanf("%d", &n);
printf("Enter the burst time and priority for each process:\n");
for(i=0; i<n; i++)
{
printf("P[%d]: ", i+1);
p[i].id = i+1;
scanf("%d %d", &p[i].burst, &p[i].priority);
}
sort(p, n);
p[0].wt = 0;
p[0].tat = p[0].burst;
for(i=1; i<n; i++)
{
p[i].wt = p[i-1].wt + p[i-1].burst;
p[i].tat = p[i].wt + p[i].burst;
}
printf("\nProcess\tBurst Time\tPriority\tTurnaround Time\tWaiting Time\n");
for(i=0; i<n; i++)
{
printf("P[%d]\t%d\t\t%d\t\t%d\t\t%d\n", p[i].id, p[i].burst, p[i].priority, p[i].tat, p[i].wt);
avg_tat += p[i].tat;
avg_wt += p[i].wt;
}
avg_tat /= n;
avg_wt /= n;
printf("\nAverage Turnaround Time: %.2f", avg_tat);
printf("\nAverage Waiting Time: %.2f", avg_wt);
return 0;
}
```
4. RR(时间片轮转调度算法)
```c
#include <stdio.h>
#include <stdlib.h>
struct process
{
int id;
int burst;
int remaining;
int tat;
int wt;
};
int main()
{
int n, i, t = 0, count = 0, tq;
float avg_tat = 0, avg_wt = 0;
struct process p[20];
printf("Enter the number of processes: ");
scanf("%d", &n);
printf("Enter the time quantum: ");
scanf("%d", &tq);
printf("Enter the burst time for each process:\n");
for(i=0; i<n; i++)
{
printf("P[%d]: ", i+1);
p[i].id = i+1;
scanf("%d", &p[i].burst);
p[i].remaining = p[i].burst;
}
while(count < n)
{
for(i=0; i<n; i++)
{
if(p[i].remaining > 0)
{
if(p[i].remaining > tq)
{
t += tq;
p[i].remaining -= tq;
}
else
{
t += p[i].remaining;
p[i].wt = t - p[i].burst;
p[i].remaining = 0;
count++;
p[i].tat = t;
avg_tat += p[i].tat;
avg_wt += p[i].wt;
}
}
}
}
avg_tat /= n;
avg_wt /= n;
printf("\nProcess\tBurst Time\tTurnaround Time\tWaiting Time\n");
for(i=0; i<n; i++)
{
printf("P[%d]\t%d\t\t%d\t\t%d\n", p[i].id, p[i].burst, p[i].tat, p[i].wt);
}
printf("\nAverage Turnaround Time: %.2f", avg_tat);
printf("\nAverage Waiting Time: %.2f", avg_wt);
return 0;
}
```
5. MFS(多级反馈队列调度算法)
```c
#include <stdio.h>
#include <stdlib.h>
struct process
{
int id;
int burst;
int remaining;
int priority;
int tat;
int wt;
};
void swap(struct process *a, struct process *b)
{
struct process temp = *a;
*a = *b;
*b = temp;
}
void sort(struct process arr[], int n)
{
int i, j;
for(i=0; i<n-1; i++)
{
for(j=0; j<n-i-1; j++)
{
if(arr[j].priority < arr[j+1].priority)
{
swap(&arr[j], &arr[j+1]);
}
}
}
}
int main()
{
int n, i, j, t = 0, count = 0;
float avg_tat = 0, avg_wt = 0;
struct process p[20];
printf("Enter the number of processes: ");
scanf("%d", &n);
printf("Enter the burst time and priority for each process:\n");
for(i=0; i<n; i++)
{
printf("P[%d]: ", i+1);
p[i].id = i+1;
scanf("%d %d", &p[i].burst, &p[i].priority);
p[i].remaining = p[i].burst;
}
sort(p, n);
while(count < n)
{
for(i=0; i<n; i++)
{
if(p[i].remaining > 0)
{
if(p[i].remaining > 2)
{
t += 2;
p[i].remaining -= 2;
p[i].priority--;
}
else
{
t += p[i].remaining;
p[i].wt = t - p[i].burst;
p[i].remaining = 0;
count++;
p[i].tat = t;
avg_tat += p[i].tat;
avg_wt += p[i].wt;
}
}
}
sort(p, n);
}
avg_tat /= n;
avg_wt /= n;
printf("\nProcess\tBurst Time\tPriority\tTurnaround Time\tWaiting Time\n");
for(i=0; i<n; i++)
{
printf("P[%d]\t%d\t\t%d\t\t%d\t\t%d\n", p[i].id, p[i].burst, p[i].priority, p[i].tat, p[i].wt);
}
printf("\nAverage Turnaround Time: %.2f", avg_tat);
printf("\nAverage Waiting Time: %.2f", avg_wt);
return 0;
}
```
阅读全文
相关推荐
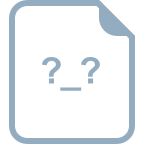
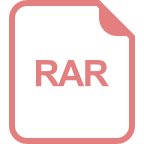
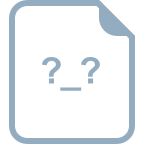
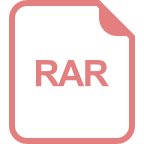
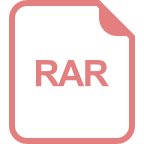
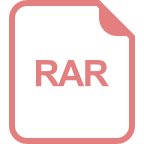
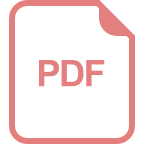
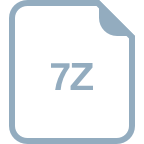
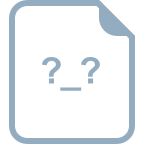
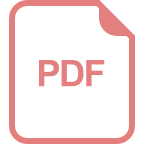
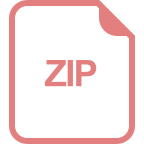
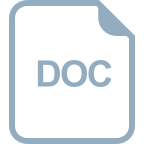
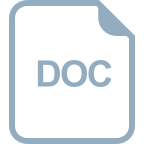
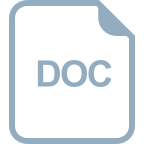
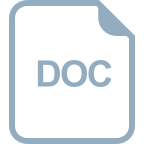
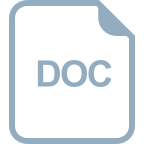
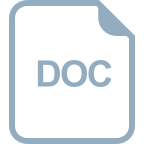
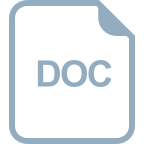