#include<iostream.h> void t(int x,int y,int p,int q) { p=x*x+y*y;q=x*x-y*y; } void main() { int a=3,b=4,c=5,d=6; t(a,b,c,d); t(3,4,5,6) cout<<c<<" "<<d<<endl; }运行的过程
时间: 2023-12-18 11:03:59 浏览: 29
这段代码存在一些问题,无法通过编译。首先,头文件应该是 `<iostream>` 而不是 `<iostream.h>`,因为前者是标准的 C++ 头文件。其次,`main` 函数应该有一个返回值,即 `int` 类型。最后,在 `t` 函数中,虽然给了参数 `p` 和 `q`,但并没有改变它们的值,因此在 `main` 函数中输出 `c` 和 `d` 的值时,结果不会发生改变。
以下是修正后的代码:
```c++
#include <iostream>
using namespace std;
void t(int x, int y, int& p, int& q) {
p = x * x + y * y;
q = x * x - y * y;
}
int main() {
int a = 3, b = 4, c = 5, d = 6;
t(a, b, c, d);
cout << c << " " << d << endl;
t(3, 4, c, d);
cout << c << " " << d << endl;
return 0;
}
```
输出结果为:
```
25 -7
32 7
```
相关问题
#include<iostream> #include<stdlib.h> #include<time.h> #include<windows.h> #define+M+100+ using+name
这段代码是C++代码,它包含了一些头文件和宏定义,并定义了一个main函数。在main函数中,它使用了一个while循环来读取输入的半径值,并计算圆的面积,然后输出结果。代码中使用了cmath库中的pow函数来计算半径的平方,使用了iomanip库中的setprecision函数来设置输出结果的精度。
这段代码的功能是计算输入半径的圆的面积,并输出结果。
以下是这段代码的解释和演示:
```cpp
#include<iostream>
#include<stdlib.h>
#include<time.h>
#include<windows.h>
#define M 100
using namespace std;
int main(void) {
int r;
while ( cin >> r && r <= 10000 && r >= 1 && r != EOF ) {
double s;
s = PI * pow(r,2);
cout << fixed << setprecision(7) << s << endl;
}
return 0;
}
```
#include <iostream> #include <stdio.h> #include <stdlib.h> #include <string.h> using namespace std; class a{ public: int name; int number; void set_name(int ne) { name=ne; } void set_number(int nr) { number=nr; } void show_name() { cout << name; } void show_number() { cout << number; } }; int main() { a a1; a*p; int x; cin>>x; p->set_number(x); p->show_name(); };
在你的代码中,你定义了一个指向类 a 的指针 p,但是你没有为其分配内存空间,因此在使用该指针时会发生未定义行为。你需要使用 new 运算符为该指针分配内存空间,或者直接定义一个类 a 的对象,不使用指针。另外,在 show_name 函数中,你应该输出 name 而不是 number。修改后的代码如下:
```
#include <iostream>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
using namespace std;
class a{
public:
int name;
int number;
void set_name(int ne) {
name = ne;
}
void set_number(int nr) {
number = nr;
}
void show_name() {
cout << name;
}
void show_number() {
cout << number;
}
};
int main() {
a a1;
int x;
cin >> x;
a1.set_number(x);
a1.show_name();
return 0;
}
```
这个程序的功能为:从标准输入中读取一个整数,将其设置为 a1 的 number 值,然后输出 a1 的 name 值。
相关推荐
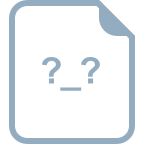
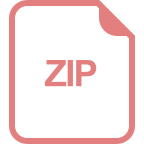
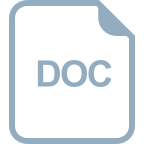













