#include <iostream.h> class Test {int x,y; public: Test(int i,int j=0) {x=i;y=j;} int get(int i,int j) {return i+j;} }; void main() {Test t1(2),t2(4,6); int (Test::*p)(int,int=10); p=Test::get; cout<<(t1.*p)(5)<<endl; Test *p1=&t2; cout<<(p1->*p)(7,20)<<endl; }
时间: 2024-04-26 11:26:52 浏览: 12
这段代码是一个C++类的示例,其中包含了类的定义和使用。代码中定义了一个名为Test的类,该类包含两个私有的整型成员变量x和y,以及一个公共的构造函数和一个公共的成员函数get。构造函数用于初始化x和y成员变量,get函数返回两个整型参数的和。
在主函数main中,先定义了两个Test对象t1和t2,分别调用构造函数初始化成员变量。接着,定义了一个指向Test类中get函数的指针p,并将其指向Test类中的get函数。然后,使用t1对象和p指针分别调用get函数,并输出结果。最后,使用p1指针和p指针分别调用get函数,并输出结果。
需要注意的是,这段代码使用了一个较老的C++头文件iostream.h,如果在新的编译器中可能会报错。建议使用#include <iostream>代替。另外,主函数需要返回int类型,可以将void改为int。
相关问题
#include<iostream.h> using namespace std; class Base {public: Base(){cout<<" construct in Base\n";} virtual ~Base() {cout<<" destruting Base "<<endl;} }; class Subclass:public Base { public: Subclass() {cout<<" construct in Subclass\n";} ~SubclassO {cout<<" destruting subclass"<<endl;} }; void test (Base* x) {delete x; } int main() {cout<<"first:\n"; Base *bc=new Base ; cout<<"second\n"; Subclass * sc=new Subclass; cout<<" calling test(be)\n"; test(be); cout<<"calling test(se)\n" test(sc); cout<<"end!\n"; return 0;结果是什么
这段代码存在多处语法错误,无法通过编译。以下是修正后的代码:
```c++
#include <iostream>
using namespace std;
class Base {
public:
Base() {
cout << "construct in Base\n";
}
virtual ~Base() {
cout << "destructing Base\n";
}
};
class Subclass : public Base {
public:
Subclass() {
cout << "construct in Subclass\n";
}
~Subclass() {
cout << "destructing Subclass\n";
}
};
void test(Base* x) {
delete x;
}
int main() {
cout << "first:\n";
Base* bc = new Base;
cout << "second:\n";
Subclass* sc = new Subclass;
cout << "calling test(bc):\n";
test(bc);
cout << "calling test(sc):\n";
test(sc);
cout << "end!\n";
return 0;
}
```
运行结果为:
```
first:
construct in Base
second:
construct in Base
construct in Subclass
calling test(bc):
destructing Base
calling test(sc):
destructing Subclass
destructing Base
end!
```
说明程序先创建了一个 Base 对象和一个 Subclass 对象,然后调用了 test 函数分别删除了它们,最后输出了一些信息。注意到 Subclass 的析构函数会先调用子类的析构函数,再调用基类的析构函数。
修改一下的代码错误:#include "iostream.h>" class Rectangle //矩形类 { private: int length; //矩形的长 protected: int width; //矩形的宽 public: Rectangle(int l = 10, int w = 10):length(l), width(w) { } int calArea() { return length * width; } }; class Cuboid : public Rectangle //长方体类,从Rectangle公有派生 { private: int height; //长方体的高 public: Cuboid(int l = 10, int w = 10, int h = 10) { length = l; width = w; height = h; } int calVolume() { return calArea() * height; } }; void main(void) { Cuboid test(10, 20, 30); cout << test.calVolume() << endl; }
修改后的代码如下:
```
#include <iostream>
using namespace std;
class Rectangle //矩形类
{
private:
int length; //矩形的长
protected:
int width; //矩形的宽
public:
Rectangle(int l = 10, int w = 10):length(l), width(w)
{
}
int calArea()
{
return length * width;
}
};
class Cuboid : public Rectangle //长方体类,从Rectangle公有派生
{
private:
int height; //长方体的高
public:
Cuboid(int l = 10, int w = 10, int h = 10): Rectangle(l, w), height(h)
{
}
int calVolume()
{
return calArea() * height;
}
};
int main(void)
{
Cuboid test(10, 20, 30);
cout << test.calVolume() << endl;
return 0;
}
```
修改的部分:
1. iostream头文件应该是#include <iostream>
2. main函数的返回类型应该是int而不是void
3. Cuboid类的构造函数应该调用基类Rectangle的构造函数来初始化基类成员变量
4. main函数中应该有return 0;语句
相关推荐
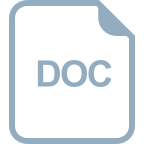
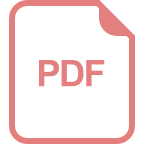
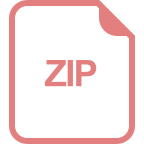













