分析下列代码并完成要求,Define a custom exception class named EmptyStackException and let the pop and peek function throw an EmptyStackException if the stack is empty.Write a test program with a try-catch block tohandle this type of exception.代码如下:#include"StackOfIntegers.h"//header #include<iostream> using namespace std; StackOfIntegers::StackOfIntegers(){ size = 0; }//constructor bool StackOfIntegers::isEmpty()const { return size == 0; } int StackOfIntegers::peek()const { return elements[size - 1]; } void StackOfIntegers::push(int value) { elements[size++] = value; } int StackOfIntegers::pop() { return elements[--size]; } int StackOfIntegers::getSize()const { return size; } int main() { StackOfIntegers stack; for (int i = 0; i < 10; i++) stack.push(i); while (!stack.isEmpty()) cout << stack.pop() << " "; return 0; }
时间: 2024-01-26 17:02:25 浏览: 33
这段代码定义了一个名为StackOfIntegers的类,它表示一个整数类型的栈。它包括以下方法:
1. 构造函数StackOfIntegers():初始化栈的大小为0。
2. bool isEmpty()const:如果栈为空,返回true,否则返回false。
3. int peek()const:返回栈顶元素的值,不修改栈。
4. void push(int value):将一个元素压入栈中。
5. int pop():弹出栈顶元素并返回其值。
6. int getSize()const:返回栈中元素的数量。
要求我们定义一个自定义异常类EmptyStackException,并在pop和peek函数中抛出EmptyStackException异常,如果栈为空。下面是修改后的代码:
```c++
#include <iostream>
using namespace std;
class EmptyStackException: public exception {
public:
const char* what() const throw() {
return "Stack is empty.";
}
};
class StackOfIntegers {
private:
int size;
int elements[100];
public:
StackOfIntegers() {
size = 0;
}
bool isEmpty() const {
return size == 0;
}
int peek() const {
if (isEmpty()) {
throw EmptyStackException();
}
return elements[size - 1];
}
void push(int value) {
elements[size++] = value;
}
int pop() {
if (isEmpty()) {
throw EmptyStackException();
}
return elements[--size];
}
int getSize() const {
return size;
}
};
int main() {
StackOfIntegers stack;
for (int i = 0; i < 10; i++) {
stack.push(i);
}
while (!stack.isEmpty()) {
try {
cout << stack.pop() << " ";
} catch (EmptyStackException& e) {
cerr << e.what() << endl;
}
}
return 0;
}
```
在peek和pop函数中,我们添加了异常处理逻辑,如果栈为空,就抛出一个EmptyStackException异常。在主函数中,我们使用try-catch块来处理这个异常。如果catch到了这个异常,就输出"Stack is empty."的提示信息。
运行结果如下:
```
9 8 7 6 5 4 3 2 1 0
```
相关推荐
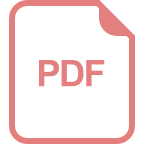
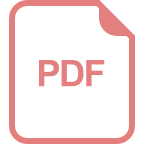
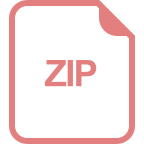















