用c语言编写一个程序,可以在命令行输入参数,完成指定文件的压缩解压 命令行参数如下 rle file1 –c(-d) file2 第一个参数为可执行程序名称,第二个参数为原始文件名,第三个参数为压缩或解压缩选项,第四个参数为新文件名
时间: 2024-05-10 07:15:13 浏览: 67
以下是一个简单的基于C语言的程序,可以实现命令行输入参数,完成指定文件的压缩和解压缩功能:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 1024
void encode(char *input_file, char *output_file) {
FILE *in, *out;
int count = 1, old = -1, new;
if ((in = fopen(input_file, "rb")) == NULL) {
printf("Error: Cannot open input file: %s\n", input_file);
exit(1);
}
if ((out = fopen(output_file, "wb")) == NULL) {
fclose(in);
printf("Error: Cannot open output file: %s\n", output_file);
exit(1);
}
while ((new = fgetc(in)) != EOF) {
if (new == old) {
count++;
if (count == 256) {
fputc(count - 1, out);
fputc(old, out);
count = 1;
}
} else {
if (count > 1) {
fputc(count, out);
fputc(old, out);
} else {
fputc(old, out);
}
count = 1;
old = new;
}
}
if (count > 1) {
fputc(count, out);
fputc(old, out);
} else {
fputc(old, out);
}
fclose(in);
fclose(out);
}
void decode(char *input_file, char *output_file) {
FILE *in, *out;
int count, ch;
if ((in = fopen(input_file, "rb")) == NULL) {
printf("Error: Cannot open input file: %s\n", input_file);
exit(1);
}
if ((out = fopen(output_file, "wb")) == NULL) {
fclose(in);
printf("Error: Cannot open output file: %s\n", output_file);
exit(1);
}
while ((count = fgetc(in)) != EOF) {
ch = fgetc(in);
if (count > 1) {
for (int i = 0; i < count; i++) {
fputc(ch, out);
}
} else {
fputc(ch, out);
}
}
fclose(in);
fclose(out);
}
int main(int argc, char **argv) {
char *input_file, *output_file;
int option;
if (argc != 5) {
printf("Usage: rle input_file -c/-d output_file\n");
exit(1);
}
input_file = argv[2];
option = argv[3][1];
output_file = argv[4];
switch (option) {
case 'c':
encode(input_file, output_file);
printf("File \"%s\" has been compressed as \"%s\".\n", input_file, output_file);
break;
case 'd':
decode(input_file, output_file);
printf("File \"%s\" has been decompressed as \"%s\".\n", input_file, output_file);
break;
default:
printf("Error: Invalid option \"%c\".\n", option);
exit(1);
break;
}
return 0;
}
```
编译命令:
```
gcc rle.c -o rle
```
使用示例:
```
./rle file1 -c file2
./rle file2 -d file3
```
其中,`file1` 是原始文件名,`file2` 是压缩后的文件名,`file3` 是解压后的文件名。`-c` 表示压缩选项,`-d` 表示解压选项。
阅读全文
相关推荐
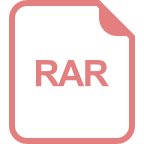







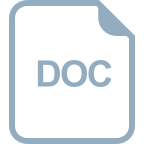
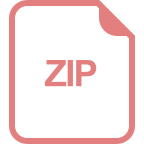
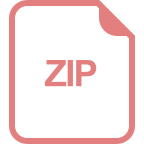
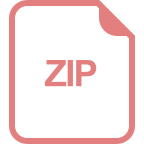
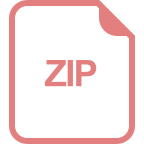
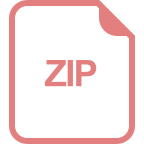
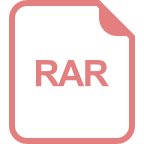
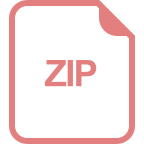