用c语言rle解压算法编写一个程序,可以在命令行输入参数,完成指定文件的压缩解压 命令行参数如下 rle file1 –c(-d) file2 第一个参数为可执行程序名称,第二个参数为原始文件名,第三个参数为压缩或解压缩选项,第四个参数为新文件名
时间: 2023-06-17 07:04:41 浏览: 59
以下是一个简单的实现,使用标准的文件操作函数和RLE算法实现压缩和解压缩。
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_COUNT 255
int main(int argc, char *argv[]) {
FILE *input_file, *output_file;
char *input_filename, *output_filename;
char current, previous;
int count;
if (argc != 4) {
printf("Usage: %s input_file -c/-d output_file\n", argv[0]);
return 1;
}
input_filename = argv[1];
output_filename = argv[3];
if ((input_file = fopen(input_filename, "rb")) == NULL) {
printf("Error: Cannot open input file %s\n", input_filename);
return 1;
}
if ((output_file = fopen(output_filename, "wb")) == NULL) {
printf("Error: Cannot open output file %s\n", output_filename);
fclose(input_file);
return 1;
}
if (argv[2][0] == '-' && argv[2][1] == 'c') {
// Compression mode
count = 0;
previous = getc(input_file);
while (!feof(input_file)) {
current = getc(input_file);
if (current == previous && count < MAX_COUNT) {
count++;
} else {
putc(count, output_file);
putc(previous, output_file);
count = 1;
previous = current;
}
}
putc(count, output_file);
putc(previous, output_file);
} else if (argv[2][0] == '-' && argv[2][1] == 'd') {
// Decompression mode
while (!feof(input_file)) {
count = getc(input_file);
current = getc(input_file);
for (int i = 0; i < count; i++) {
putc(current, output_file);
}
}
} else {
printf("Error: Invalid option %s\n", argv[2]);
fclose(input_file);
fclose(output_file);
return 1;
}
fclose(input_file);
fclose(output_file);
return 0;
}
```
使用方法:
将上述代码保存为`rle.c`,然后在命令行中执行以下命令:
```
gcc rle.c -o rle
./rle file1 -c file2 # 压缩 file1 到 file2
./rle file2 -d file3 # 解压 file2 到 file3
```
相关推荐
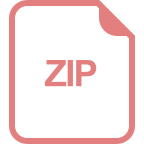
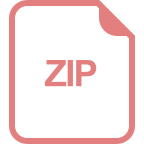











