用c语言编写一个程序,可以再命令行输入参数,实现对指定文件的压缩解压。命令行参数如下:rle file1 -c(-d)file2 第一个参数为可执行程序名称,第二个参数为原始文件名,第三个参数为压缩或解压缩选项,第四个参数为新文件名
时间: 2023-07-15 20:11:56 浏览: 100
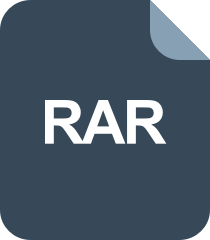
C语言 文件压缩和解压

以下是一个使用C语言编写的实现对指定文件的压缩解压的程序。该程序可以通过命令行参数来指定要压缩或解压的文件和选项,以及新文件的命名。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_FILENAME_LENGTH 256
#define MAX_FILESIZE 1000000
void compress(char *in_filename, char *out_filename);
void decompress(char *in_filename, char *out_filename);
int main(int argc, char *argv[]) {
char in_filename[MAX_FILENAME_LENGTH];
char out_filename[MAX_FILENAME_LENGTH];
char option;
if (argc != 5) {
printf("Usage: %s <input file> -c/-d <output file>\n", argv[0]);
return 0;
}
strncpy(in_filename, argv[1], MAX_FILENAME_LENGTH);
option = argv[2][1];
strncpy(out_filename, argv[4], MAX_FILENAME_LENGTH);
if (option == 'c') {
compress(in_filename, out_filename);
} else if (option == 'd') {
decompress(in_filename, out_filename);
}
return 0;
}
void compress(char *in_filename, char *out_filename) {
FILE *in_file, *out_file;
unsigned char buffer[MAX_FILESIZE];
unsigned char compressed_buffer[MAX_FILESIZE];
unsigned char current_char;
int buffer_size = 0;
int compressed_size = 0;
int i, j;
in_file = fopen(in_filename, "rb");
if (in_file == NULL) {
printf("Error opening input file\n");
return;
}
out_file = fopen(out_filename, "wb");
if (out_file == NULL) {
printf("Error opening output file\n");
fclose(in_file);
return;
}
while ((current_char = fgetc(in_file)) != EOF) {
if (buffer_size == 0 || buffer[buffer_size - 1] != current_char) {
buffer[buffer_size++] = current_char;
} else {
int count = 1;
while (count <= 255 && (current_char = fgetc(in_file)) != EOF && current_char == buffer[buffer_size - 1]) {
count++;
}
buffer[buffer_size++] = count;
buffer[buffer_size++] = current_char;
}
}
for (i = 0; i < buffer_size; i++) {
if (i % 2 == 0) {
compressed_buffer[compressed_size++] = buffer[i];
} else {
for (j = 0; j < buffer[i]; j++) {
compressed_buffer[compressed_size++] = buffer[i + 1];
}
i++;
}
}
fwrite(compressed_buffer, 1, compressed_size, out_file);
fclose(in_file);
fclose(out_file);
}
void decompress(char *in_filename, char *out_filename) {
FILE *in_file, *out_file;
unsigned char buffer[MAX_FILESIZE];
unsigned char decompressed_buffer[MAX_FILESIZE];
unsigned char current_char;
int buffer_size = 0;
int decompressed_size = 0;
int i;
in_file = fopen(in_filename, "rb");
if (in_file == NULL) {
printf("Error opening input file\n");
return;
}
out_file = fopen(out_filename, "wb");
if (out_file == NULL) {
printf("Error opening output file\n");
fclose(in_file);
return;
}
fread(buffer, 1, MAX_FILESIZE, in_file);
for (i = 0; i < MAX_FILESIZE && buffer[i] != 0; i++) {
if (i % 2 == 0) {
decompressed_buffer[decompressed_size++] = buffer[i];
} else {
int count = buffer[i];
while (count-- > 0) {
decompressed_buffer[decompressed_size++] = buffer[i + 1];
}
i++;
}
}
fwrite(decompressed_buffer, 1, decompressed_size, out_file);
fclose(in_file);
fclose(out_file);
}
```
程序中使用了两个函数:`compress`和`decompress`。`compress`函数将输入文件压缩并写入输出文件,`decompress`函数将压缩后的文件解压并写入输出文件。
在`main`函数中,程序首先检查命令行参数的数量是否正确。如果不正确,则输出用法信息并退出程序。如果参数数量正确,则将输入文件名、选项和输出文件名分别存储在相应的变量中。
根据选项的不同,程序调用`compress`或`decompress`函数来执行相应的操作。在这两个函数中,程序使用标准C文件操作函数(例如`fopen`、`fread`、`fwrite`等)来读取或写入文件。
请注意,此程序是为了演示目的而编写的,并不一定适用于所有情况。例如,程序没有处理文件过大的情况,也没有进行错误处理。在实际项目中使用此程序时,请务必进行适当的修改和测试。
阅读全文
相关推荐
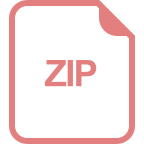







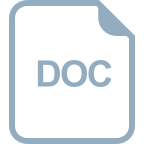
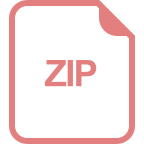
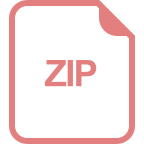
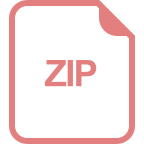
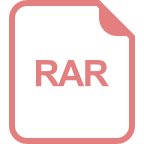
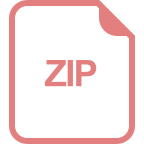
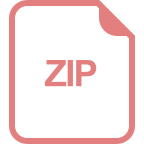
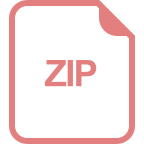
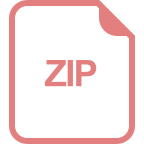