Python pyqt5 写一个登陆界面
时间: 2024-03-06 14:20:54 浏览: 23
好的,您可以按照以下步骤使用Python和PyQt5编写一个简单的登录界面:
1. 首先,导入必要的PyQt5模块:
```
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QLineEdit, QPushButton
```
2. 创建一个QWidget窗口对象,并设置窗口的大小和标题:
```
app = QApplication([])
window = QWidget()
window.setWindowTitle("登录")
window.resize(300, 150)
```
3. 在窗口上添加标签、文本框和按钮等控件:
```
label_username = QLabel('用户名:', window)
label_username.move(20, 20)
text_username = QLineEdit(window)
text_username.move(100, 20)
label_password = QLabel('密码:', window)
label_password.move(20, 50)
text_password = QLineEdit(window)
text_password.setEchoMode(QLineEdit.Password)
text_password.move(100, 50)
btn_login = QPushButton('登录', window)
btn_login.move(100, 100)
```
4. 定义按钮点击事件,判断输入的用户名和密码是否正确:
```
def login():
username = text_username.text().strip()
password = text_password.text().strip()
if not username:
label_username.setText('<font color="red">用户名不能为空</font>')
return
if not password:
label_password.setText('<font color="red">密码不能为空</font>')
return
if username == 'admin' and password == 'admin':
label_username.setText('')
label_password.setText('')
print('登录成功')
else:
label_username.setText('<font color="red">用户名或密码错误</font>')
label_password.setText('')
btn_login.clicked.connect(login)
```
5. 最后,显示窗口并运行Qt应用程序:
```
window.show()
app.exec_()
```
完整的代码如下所示:
```
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QLineEdit, QPushButton
app = QApplication([])
window = QWidget()
window.setWindowTitle("登录")
window.resize(300, 150)
label_username = QLabel('用户名:', window)
label_username.move(20, 20)
text_username = QLineEdit(window)
text_username.move(100, 20)
label_password = QLabel('密码:', window)
label_password.move(20, 50)
text_password = QLineEdit(window)
text_password.setEchoMode(QLineEdit.Password)
text_password.move(100, 50)
btn_login = QPushButton('登录', window)
btn_login.move(100, 100)
def login():
username = text_username.text().strip()
password = text_password.text().strip()
if not username:
label_username.setText('<font color="red">用户名不能为空</font>')
return
if not password:
label_password.setText('<font color="red">密码不能为空</font>')
return
if username == 'admin' and password == 'admin':
label_username.setText('')
label_password.setText('')
print('登录成功')
else:
label_username.setText('<font color="red">用户名或密码错误</font>')
label_password.setText('')
btn_login.clicked.connect(login)
window.show()
app.exec_()
```
希望这可以回答您的问题,如果您有任何其他问题,请继续提问。
相关推荐
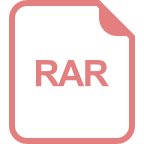
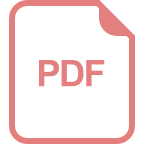














