用python反转链表并打印原链表和反转后的链表
时间: 2024-09-19 20:08:31 浏览: 44
在Python中,反转链表是一个常见的链表操作。首先,我们需要创建两个指针,一个当前节点,一个前驱节点。然后我们遍历链表,每次将当前节点的下一个节点赋值给当前节点,同时将当前节点移动到下一个位置,直到遍历完整个链表。最后,我们将前驱节点设为原始头节点,得到的就是反转后的链表。
以下是简单的代码示例:
```python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_list(head):
prev = None
current = head
while current is not None:
# 反转节点的指向
temp = current.next
current.next = prev
# 更新前驱和当前位置
prev = current
current = temp
return prev
# 假设有一个链表
original_list = ListNode(1, ListNode(2, ListNode(3, ListNode(4, ListNode(5)))))
# 打印原链表
def print_list(head):
while head:
print(head.val, end=" -> ")
head = head.next
print("None")
# 反转链表
reversed_head = reverse_list(original_list)
# 打印反转后的链表
print("Reversed list:")
print_list(reversed_head)
```
运行这个代码,你会看到原链表和反转后的链表的输出结果。
阅读全文
相关推荐
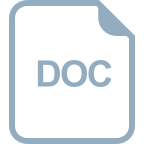
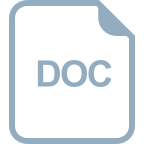
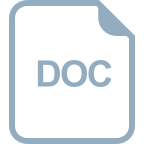














