应用 c语言编写一个线性表操作测试程序。程序中用户可以选择插入数据元素,查找数 据元素,删除数据元素,及显示数据元素。程序启动后,于屏幕上显示如下功能菜单。 线性表基本操作实验 1、输入元素 2、查看
时间: 2024-10-06 14:02:48 浏览: 46
在C语言中,我们可以创建一个简单的线性表操作测试程序,比如使用结构体作为节点,数组或链表来存储数据。以下是程序的一个基础框架:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义线性表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode)
return NULL;
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 插入元素到列表
void insertElement(Node** head, int value) {
Node* newNode = createNode(value);
if (*head == NULL) {
*head = newNode;
} else {
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 查找元素
int searchElement(Node* head, int value) {
Node* current = head;
while (current != NULL) {
if (current->data == value)
return 1; // 成功找到
current = current->next;
}
return 0; // 未找到
}
// 删除元素
void deleteElement(Node** head, int value) {
if (*head == NULL)
return;
if ((*head)->data == value) {
Node* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
Node* current = *head;
while (current->next != NULL && current->next->data != value) {
current = current->next;
}
if (current->next != NULL)
current->next = current->next->next;
free(current->next); // 释放已删除节点的内存
}
// 显示所有元素
void displayList(Node* head) {
Node* current = head;
printf("Elements in the list: ");
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
Node* head = NULL;
char choice;
do {
printf("\nLinear List Operations:\n1. Insert\n2. Search\n3. Delete\n4. Display\n5. Exit\nEnter your choice: ");
scanf("%c", &choice);
switch (choice) {
case '1':
int value;
printf("Enter a value to insert: ");
scanf("%d", &value);
insertElement(&head, value);
break;
case '2':
int searchVal;
printf("Enter a value to search: ");
scanf("%d", &searchVal);
int found = searchElement(head, searchVal);
if (found)
printf("Value found.\n");
else
printf("Value not found.\n");
break;
// 其他选项添加类似处理...
case '5':
printf("Exiting...\n");
break;
default:
printf("Invalid choice. Please try again.\n");
break;
}
} while (choice != '5');
return 0;
}
```
这个程序实现了基本的线性表操作,包括插入、搜索、删除和显示。用户可以根据提示选择相应的操作。
阅读全文
相关推荐
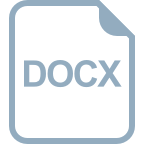
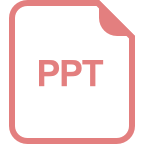
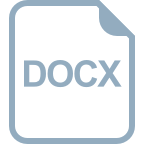
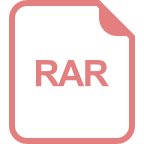
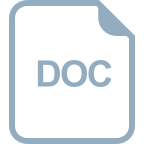













