pytorch实现MAE损失
时间: 2023-07-17 17:06:23 浏览: 132
在 PyTorch 中,可以使用以下代码实现均方误差(Mean Absolute Error,MAE)损失函数:
```python
import torch
import torch.nn.functional as F
def mae_loss(output, target):
loss = F.l1_loss(output, target)
return loss
```
在上述代码中,我们使用了 PyTorch 提供的 `F.l1_loss` 函数来计算 MAE 损失。`F.l1_loss` 函数计算了输出值 `output` 和目标值 `target` 之间的平均绝对误差。
你可以将上述代码嵌入到你的模型训练循环中,并将生成值和目标值传递给 `mae_loss` 函数来计算 MAE 损失。最后,你可以使用反向传播和优化器来更新模型的参数,以最小化 MAE 损失。
需要注意的是,MAE 损失函数对于异常值更加鲁棒,因为它不会放大较大的误差。因此,在某些情况下,MAE 可能比均方误差(MSE)更适合作为损失函数。
相关问题
pytorch实现MAE损失函数
PyTorch中可以使用torch.nn.L1Loss来实现MAE(Mean Absolute Error)损失函数。该函数的定义如下:class torch.nn.L1Loss(size_average=None, reduce=None, reduction='mean')。你可以直接调用该函数来计算MAE损失。
pytorch的MAE损失函数
PyTorch中的MAE(Mean Absolute Error)损失函数用于衡量预测值与真实值之间的平均绝对误差。它是回归问题中常用的损失函数之一。
在PyTorch中,可以使用`torch.nn.L1Loss`类来实现MAE损失函数。该类计算的是预测值与真实值之间的平均绝对误差。
以下是使用PyTorch中的MAE损失函数的示例代码:
```python
import torch
import torch.nn as nn
# 创建预测值和真实值
predictions = torch.tensor([2.5, 3.7, 4.1, 5.2])
targets = torch.tensor([2.0, 4.0, 4.5, 5.0])
# 创建MAE损失函数对象
mae_loss = nn.L1Loss()
# 计算MAE损失
loss = mae_loss(predictions, targets)
print(loss.item()) # 打印损失值
```
输出结果为:
```
0.4750000238418579
```
相关推荐
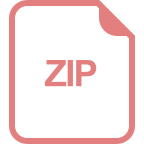
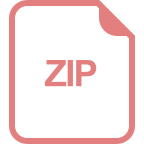












