基于rs485的简单现场总线通信系统单发单收c语言程序
时间: 2023-09-20 07:03:43 浏览: 38
以下是基于RS485的简单现场总线通信系统的单发单收C语言程序示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <termios.h>
#define BAUDRATE B9600
#define MODEMDEVICE "/dev/ttyS0"
#define _POSIX_SOURCE 1
int set_interface_attribs(int fd, int speed)
{
struct termios tty;
if (tcgetattr(fd, &tty) < 0) {
printf("Error from tcgetattr: %s\n", strerror(errno));
return -1;
}
cfsetospeed(&tty, (speed_t)speed);
cfsetispeed(&tty, (speed_t)speed);
tty.c_cflag |= (CLOCAL | CREAD);
tty.c_cflag &= ~CSIZE;
tty.c_cflag |= CS8;
tty.c_cflag &= ~PARENB;
tty.c_cflag &= ~CSTOPB;
tty.c_cflag &= ~CRTSCTS;
tty.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
tty.c_iflag &= ~(IXON | IXOFF | IXANY);
tty.c_iflag &= ~(IGNBRK|BRKINT|PARMRK|ISTRIP|INLCR|IGNCR|ICRNL);
tty.c_oflag &= ~OPOST;
tty.c_cc[VTIME] = 0;
tty.c_cc[VMIN] = 1;
if (tcsetattr(fd, TCSANOW, &tty) != 0) {
printf("Error from tcsetattr: %s\n", strerror(errno));
return -1;
}
return 0;
}
void set_blocking(int fd, int should_block)
{
struct termios tty;
memset(&tty, 0, sizeof(tty));
if (tcgetattr(fd, &tty) != 0) {
printf("Error from tggetattr: %s\n", strerror(errno));
return;
}
tty.c_cc[VMIN] = should_block ? 1 : 0;
tty.c_cc[VTIME] = 5;
if (tcsetattr(fd, TCSANOW, &tty) != 0)
printf("Error setting term attributes: %s\n", strerror(errno));
}
int main(int argc, char *argv[])
{
int fd;
char buf[256];
char *portname = MODEMDEVICE;
if (argc > 1) {
portname = argv[1];
}
fd = open(portname, O_RDWR | O_NOCTTY | O_SYNC);
if (fd < 0) {
printf("Error opening %s: %s\n", portname, strerror(errno));
return -1;
}
set_interface_attribs(fd, BAUDRATE);
set_blocking(fd, 0);
// 发送数据
char send_buf[] = "Hello, RS485!";
write(fd, send_buf, sizeof(send_buf));
// 接收数据
int n = read(fd, buf, sizeof(buf));
if (n > 0) {
buf[n] = 0;
printf("Received %d bytes: %s\n", n, buf);
}
close(fd);
return 0;
}
```
在这里,我们使用了Linux下的串口通信库`termios`。`set_interface_attribs`函数设置了串口的参数,包括波特率、数据位、停止位等。`set_blocking`函数设置了串口的阻塞方式,如果`should_block`为1表示阻塞读取,否则表示非阻塞读取。在`main`函数中,我们先打开串口设备,然后发送一条数据,再接收一条数据。注意,在RS485总线上,需要在发送和接收数据前进行总线控制,具体方法可以参考RS485总线的通信协议。
相关推荐
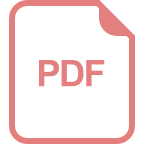
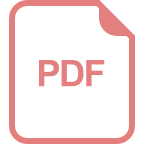














