pandas将列表中的数据写入excel的指定列中
时间: 2023-10-03 13:10:26 浏览: 64
可以使用 pandas 库中的 `DataFrame` 和 `ExcelWriter` 对象来实现将列表中的数据写入 excel 的指定列中。具体操作如下:
1. 导入 pandas 库:
```python
import pandas as pd
```
2. 创建一个 `DataFrame` 对象:
```python
df = pd.DataFrame(columns=['列名'])
```
其中,`columns` 参数指定了该 `DataFrame` 的列名,可以根据实际需求进行修改。
3. 将列表中的数据添加到 `DataFrame` 中指定的列中:
```python
df['列名'] = 列表数据
```
其中,`列名` 参数指定了要写入的列名,`列表数据` 参数指定了要写入的数据,可以根据实际需求进行修改。
4. 创建一个 `ExcelWriter` 对象:
```python
writer = pd.ExcelWriter('文件名.xlsx', engine='xlsxwriter')
```
其中,`文件名.xlsx` 参数指定了要写入的 excel 文件名。
5. 将 `DataFrame` 中的数据写入到 excel 文件中指定的列中:
```python
df.to_excel(writer, sheet_name='Sheet1', startcol=0, index=False)
```
其中,`writer` 参数指定了要写入的 excel 文件,`sheet_name` 参数指定了要写入的 sheet 名称,`startcol` 参数指定了要写入数据的起始列,`index` 参数指定了是否要将 `DataFrame` 的 index 写入到 excel 中,可以根据实际需求进行修改。
6. 保存并关闭 `ExcelWriter` 对象:
```python
writer.save()
writer.close()
```
完整代码示例:
```python
import pandas as pd
# 创建 DataFrame 对象
df = pd.DataFrame(columns=['列名'])
# 将列表中的数据添加到 DataFrame 中指定的列中
df['列名'] = 列表数据
# 创建 ExcelWriter 对象
writer = pd.ExcelWriter('文件名.xlsx', engine='xlsxwriter')
# 将 DataFrame 中的数据写入到 excel 文件中指定的列中
df.to_excel(writer, sheet_name='Sheet1', startcol=0, index=False)
# 保存并关闭 ExcelWriter 对象
writer.save()
writer.close()
```
相关推荐
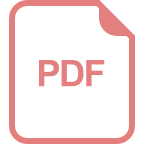
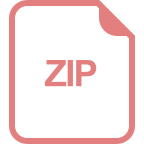
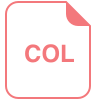
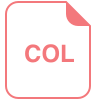
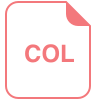
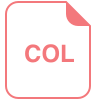
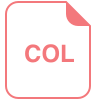









