我希望你写一个基于MINIST数据集的神经网络,使用Pycharm,实现手写数字分类,不要解释,给出代码
时间: 2023-02-14 17:23:51 浏览: 82
```python
import tensorflow as tf
from tensorflow import keras
# 导入MINIST数据集
(x_train, y_train), (x_test, y_test) = keras.datasets.mnist.load_data()
# 数据预处理
x_train = x_train / 255.0
x_test = x_test / 255.0
# 构建模型
model = keras.Sequential([
keras.layers.Flatten(input_shape=(28, 28)),
keras.layers.Dense(128, activation='relu'),
keras.layers.Dense(10, activation='softmax')
])
# 编译模型
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# 训练模型
model.fit(x_train, y_train, epochs=5)
# 测试模型
test_loss, test_acc = model.evaluate(x_test, y_test, verbose=2)
print('\nTest accuracy:', test_acc)
```
相关问题
我希望你写一个基于MINIST数据集的神经网络,使用Pycharm,实现手写数字分类
### 回答1:
可以使用Pycharm来实现基于MINST数据集的神经网络,用于手写数字分类。 你需要首先安装Pycharm,然后创建一个新项目。接着,你需要导入MINST数据集,可以使用Tensorflow或者Keras来载入数据。然后,您可以使用这些库来构建神经网络模型,并使用训练数据进行训练。最后,您可以使用测试数据来评估模型的准确性。
### 回答2:
首先,使用MINST数据集进行手写数字分类的任务是一个经典的机器学习问题。 MINST数据集包含了来自于大量不同人手写的0到9之间的数字图片。为了实现这个任务,我们可以使用Pycharm来编写神经网络模型。
首先,我们需要导入所需的库和模块,包括numpy、tensorflow和matplotlib等。然后,我们可以使用tensorflow提供的API加载MINST数据集,并分为训练集和测试集。
接下来,我们可以定义一个多层感知机(MLP)模型来进行手写数字分类。这里我们可以使用tensorflow提供的神经网络API,如`tf.keras.models.Sequential`和`tf.keras.layers.Dense`等来构建我们的模型。模型可以包含多个隐藏层,每个隐藏层可以包含任意数量的神经元。最后一层是一个softmax激活函数的全连接层,用于输出0到9之间的数字的概率分布。
然后,我们可以选择适当的优化算法,如随机梯度下降(SGD)或Adam,以及合适的损失函数,如交叉熵损失函数。我们可以使用`model.compile`来配置模型的优化器和损失函数。
接下来,我们可以使用训练集数据来训练我们的模型。可以使用`model.fit`来进行模型训练,指定训练数据和标签、批次大小、迭代次数等参数。
最后,我们可以使用测试集数据评估我们训练好的模型。通过使用`model.evaluate`函数,传入测试集数据和标签,模型将输出测试集上的准确率等评估指标。
在模型训练和评估之后,我们还可以使用模型对新的手写数字进行分类预测。我们可以选择任意一张手写数字图片,并将其转换为模型可接受的输入格式(如将其转换为numpy数组),然后使用`model.predict`来获得预测结果。
总之,使用Pycharm编写基于MINIST数据集的神经网络模型并实现手写数字分类可以按照上述步骤进行。通过合适选择神经网络结构、优化算法和损失函数等配置,并充分地训练和评估模型,我们可以得到一个准确率较高的手写数字分类器。
### 回答3:
首先,在PyCharm中创建一个新的Python项目。然后,我们需要导入需要的库,包括tensorflow、keras和numpy。在PyCharm中,可以使用以下命令来安装这些库:
```
pip install tensorflow
pip install keras
pip install numpy
```
接下来,我们需要下载并加载MINIST数据集。我们可以使用以下代码来下载和加载数据集:
```python
from keras.datasets import mnist
# 加载MNIST数据集
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# 数据预处理
x_train = x_train.reshape(x_train.shape[0], 28, 28, 1)
x_test = x_test.reshape(x_test.shape[0], 28, 28, 1)
x_train = x_train.astype('float32')
x_test = x_test.astype('float32')
x_train /= 255
x_test /= 255
```
接着,我们可以定义神经网络的模型。在这个示例中,我们将使用一个卷积神经网络(CNN)模型。代码如下:
```python
from keras.models import Sequential
from keras.layers import Dense, Conv2D, MaxPooling2D, Flatten
# 定义模型
model = Sequential()
model.add(Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(28, 28, 1)))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Flatten())
model.add(Dense(64, activation='relu'))
model.add(Dense(10, activation='softmax'))
# 编译模型
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
```
现在,我们可以使用训练数据来训练我们的模型。代码如下:
```python
from keras.utils import np_utils
# 将类别转换为one-hot编码
y_train = np_utils.to_categorical(y_train, 10)
y_test = np_utils.to_categorical(y_test, 10)
# 训练模型
model.fit(x_train, y_train, batch_size=128, epochs=10, verbose=1)
```
最后,我们可以使用测试数据对我们的模型进行评估,并输出准确率。代码如下:
```python
# 评估模型
score = model.evaluate(x_test, y_test, verbose=0)
print('Test accuracy:', score[1])
```
以上就是一个基于MINIST数据集的神经网络,使用PyCharm实现手写数字分类的简单示例。这只是一个基本的示例,你可以根据自己的需求和扩展定制和调整神经网络模型的结构和参数来提高准确率。
我希望你写一个基于MINIST数据集的神经网络,使用pytorch,实现手写数字分类。我希望有完整的代码结构,并输出测试结果。不要解释,给出代码
```
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision import datasets, transforms
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.conv1 = nn.Conv2d(1, 32, 3, 1)
self.conv2 = nn.Conv2d(32, 64, 3, 1)
self.dropout1 = nn.Dropout2d(0.25)
self.dropout2 = nn.Dropout2d(0.5)
self.fc1 = nn.Linear(9216, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = self.conv1(x)
x = torch.relu(x)
x = self.conv2(x)
x = torch.relu(x)
x = torch.max_pool2d(x, 2)
x = self.dropout1(x)
x = torch.flatten(x, 1)
x = self.fc1(x)
x = torch.relu(x)
x = self.dropout2(x)
x = self.fc2(x)
output = torch.log_softmax(x, dim=1)
return output
def train(model, device, train_loader, optimizer, epoch):
model.train()
for batch_idx, (data, target) in enumerate(train_loader):
data, target = data.to(device), target.to(device)
optimizer.zero_grad()
output = model(data)
loss = torch.nn.functional.nll_loss(output, target)
loss.backward()
optimizer.step()
print('Train Epoch: {} \tLoss: {:.6f}'.format(epoch, loss.item()))
def test(model, device, test_loader):
model.eval()
test_loss = 0
correct = 0
with torch.no_grad():
for data, target in test_loader:
data, target = data.to(device), target.to(device)
output = model(data)
test_loss += torch.nn.functional.nll_loss(output, target, reduction='sum').item() # sum up batch loss
pred = output.argmax(dim=1, keepdim=True) # get the index of the max log-probability
correct += pred.eq(target.view_as(pred)).sum().item()
test_loss /= len(test_loader.dataset)
print('Test set: Average loss: {:.4f}, Accuracy: {}/{} ({:.0f}%)\n'.format(test_loss, correct, len(test_loader.dataset), 100. * correct / len(test_loader.dataset)))
if __name__ == '__main__':
torch.manual_seed(1)
device = torch.device("cpu")
kwargs = {'num_workers':
相关推荐
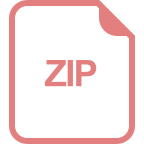
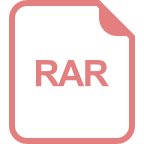
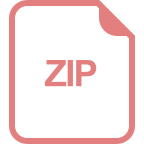












