c语音读取json文件进行解析
时间: 2023-06-21 08:10:47 浏览: 57
要读取和解析 JSON 文件,可以使用 C 语言中的第三方库 cJSON。这个库提供了一些简单的 API 来读取 JSON 文件并将其转换为 C 语言中的数据结构。
以下是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include "cJSON.h"
int main() {
// 读取 JSON 文件内容
FILE *file = fopen("example.json", "r");
if (!file) {
printf("Failed to open file\n");
return -1;
}
fseek(file, 0, SEEK_END);
long file_size = ftell(file);
fseek(file, 0, SEEK_SET);
char *json_str = (char *) malloc(file_size + 1);
fread(json_str, 1, file_size, file);
fclose(file);
json_str[file_size] = '\0';
// 解析 JSON 内容
cJSON *root = cJSON_Parse(json_str);
if (!root) {
printf("Failed to parse JSON\n");
free(json_str);
return -1;
}
// 访问 JSON 内容
cJSON *name = cJSON_GetObjectItem(root, "name");
if (name) {
printf("Name: %s\n", name->valuestring);
} else {
printf("Name not found\n");
}
cJSON *age = cJSON_GetObjectItem(root, "age");
if (age) {
printf("Age: %d\n", age->valueint);
} else {
printf("Age not found\n");
}
// 释放资源
cJSON_Delete(root);
free(json_str);
return 0;
}
```
在这个例子中,我们打开一个名为 `example.json` 的 JSON 文件,读取其内容并解析为一个 cJSON 对象。然后,我们使用 cJSON 提供的 API 访问 JSON 内容。最后,我们释放所有分配的内存并关闭文件。
需要注意的是,这个例子中只演示了如何读取和解析一个简单的 JSON 文件。如果 JSON 文件包含嵌套结构和数组等复杂内容,需要使用 cJSON 提供的更多 API 来访问这些内容。
相关推荐
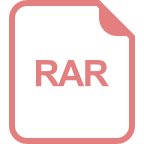













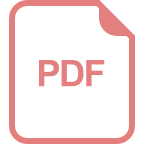