from pyspark.ml.feature import StringIndexer, VectorAssembler from pyspark.ml.regression import LinearRegression from pyspark.ml.evaluation import RegressionEvaluator from pyspark.sql import SparkSession spark = SparkSession.builder.appName("DataPrediction").getOrCreate() # 加载数据 data = spark.read.csv("graduate.csv", header=True, inferSchema=True) # 对分类变量进行索引 gender_indexer = StringIndexer(inputCol="sex", outputCol="gender_index") major_indexer = StringIndexer(inputCol="major", outputCol="major_index") hobby_indexer = StringIndexer(inputCol="hobby", outputCol="hobby_index") # 将所有特征列合并成一个向量 assembler = VectorAssembler(inputCols=["gender_index", "major_index", "hobby_index", "salary"], outputCol="features") # 划分数据集为训练集和测试集 train_data, test_data = data.randomSplit([0.7, 0.3], seed=123) # 创建线性回归模型 lr = LinearRegression(featuresCol="features", labelCol="grade") # 训练模型 model = lr.fit(train_data) # 在测试集上进行预测 predictions = model.transform(test_data) # 评估模型 evaluator = RegressionEvaluator(labelCol="grade", predictionCol="prediction", metricName="rmse") rmse = evaluator.evaluate(predictions) print("Root Mean Squared Error (RMSE) on test data = %g" % rmse) 该段代码为什么会出现pyspark.sql.utils.IllegalArgumentException: 'Field "features" does not exist.\nAvailable fields: id, grade, sex, major, salary, hobby'错误
时间: 2024-03-15 14:43:35 浏览: 14
这个错误可能是由于 `VectorAssembler` 没有成功创建 `features` 列导致的。你可以检查以下几个可能的原因:
1. `VectorAssembler` 中输入列的名称是否与数据集中的列名匹配。请确保输入列的名称和数据集中的列名完全匹配,包括大小写和空格。
2. 在创建 `VectorAssembler` 后,是否有对数据集进行缺失值处理。如果数据集中有缺失值,则会导致无法创建 `features` 列。
3. `train_data` 和 `test_data` 中是否存在 `features` 列。请确保在划分数据集时,使用了正确的数据集和列名。
你可以逐一排除以上原因,以确定是哪个问题导致了该错误。
相关问题
from pyspark.mllib.evaluation import BinaryClassificationMetrics from pyspark.sql import SparkSession from pyspark.ml.feature import VectorAssembler, StandardScaler from pyspark.ml.classification import LogisticRegression from pyspark.ml.evaluation import BinaryClassificationEvaluator
这段代码是导入所需的Spark MLlib和Spark SQL模块中的相关类和函数。具体说明如下:
- `BinaryClassificationMetrics`:Spark MLlib中二分类模型的评估指标类;
- `SparkSession`:Spark SQL中创建SparkSession的类;
- `VectorAssembler`:Spark MLlib中特征向量转换器的类,用于将多个特征列合并成一个特征向量列;
- `StandardScaler`:Spark MLlib中特征标准化类,用于对特征向量进行标准化处理;
- `LogisticRegression`:Spark MLlib中逻辑回归算法的类;
- `BinaryClassificationEvaluator`:Spark MLlib中二分类模型的评估器类,用于评估模型的性能。
这些类和函数是机器学习中常用的工具,用于对数据进行预处理、训练模型和评估模型性能。在使用这些类和函数之前,需要先导入相应的模块。
pyspark环境中 name 'LabeledPoint' is not defined
这个错误通常是因为没有正确导入 `LabeledPoint` 类。`LabeledPoint` 是 `pyspark.ml.linalg` 模块中的一个类,用于表示带有标签的向量数据。
你可以尝试在代码中添加以下导入语句:
```python
from pyspark.mllib.regression import LabeledPoint
```
如果你使用的是 `pyspark.ml` 模块而不是 `pyspark.mllib` 模块,那么你需要使用以下导入语句:
```python
from pyspark.ml.linalg import Vectors
from pyspark.ml.feature import StringIndexer
from pyspark.ml.classification import LogisticRegression
from pyspark.ml.evaluation import MulticlassClassificationEvaluator
from pyspark.ml.feature import VectorAssembler
from pyspark.ml.classification import RandomForestClassifier
from pyspark.ml.classification import DecisionTreeClassifier
from pyspark.ml.classification import GBTClassifier
from pyspark.ml.classification import NaiveBayes
from pyspark.ml.classification import LinearSVC
from pyspark.ml.classification import OneVsRest
from pyspark.ml.classification import MultilayerPerceptronClassifier
```
这些导入语句应该能够解决 `LabeledPoint` 未定义的问题。
相关推荐
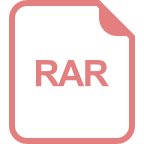
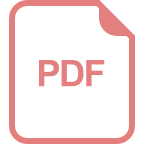













