帮我用python写一个yolov5
时间: 2023-09-03 15:27:12 浏览: 126
### 回答1:
好的,这是一个使用 YOLOv5 的简单 Python 例子:
```
import cv2
import numpy as np
# 载入 YOLOv5 预训练模型
model = cv2.dnn.readNetFromDarknet("yolov5.cfg", "yolov5.weights")
# 读入图片并调整大小
image = cv2.imread("image.jpg")
image = cv2.resize(image, (640, 480))
# 获取图片信息
(H, W) = image.shape[:2]
# 建立一个 blob,输入网络中
blob = cv2.dnn.blobFromImage(image, 1 / 255.0, (416, 416),
swapRB=True, crop=False)
# 输入网络
model.setInput(blob)
# 获取输出
layer_names = model.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in model.getUnconnectedOutLayers()]
predictions = model.forward(output_layers)
# 遍历所有的预测结果
for i in range(len(predictions)):
# 获取置信度最高的那一项
confidence = predictions[i][0][0][2]
# 确保置信度足够高
if confidence > 0.5:
# 计算坐标
x1 = int(predictions[i][0][0][0] * W)
y1 = int(predictions[i][0][0][1] * H)
x2 = int(predictions[i][0][0][2] * W)
y2 = int(predictions[i][0][0][3] * H)
# 在图片上绘制矩形
cv2.rectangle(image, (x1, y1), (x2, y2), (0, 255, 0), 2)
# 显示图片
cv2.imshow("Image", image)
cv2.waitKey(0)
```
希望这对你有帮助!
### 回答2:
当然可以!YOLOv5是一个非常流行的目标检测算法,并且可以用Python来实现。下面是一个基本的代码示例:
首先,你需要安装yolov5库。你可以使用以下命令进行安装:
```
pip install yolov5[torch]
```
接下来,创建一个Python文件,比如 "yolov5_detection.py"。在文件中,你需要导入所需的库和模块:
```python
import torch
from PIL import Image
from torchvision.transforms import ToTensor
from IPython.display import display
from models.experimental import attempt_load
from utils.general import non_max_suppression, scale_coords
from utils.torch_utils import select_device, time_synchronized
```
然后,你需要定义一些辅助函数来加载模型和进行目标检测:
```python
def load_model(model_file):
device = select_device('')
model = attempt_load(model_file, map_location=device) # 加载模型并指定设备
return model, device
def detect_objects(image, model, device):
image_tensor = ToTensor()(image).unsqueeze(0).to(device)
model.eval()
with torch.no_grad():
pred = model(image_tensor) # 进行目标检测
pred = non_max_suppression(pred, conf_thres=0.5, iou_thres=0.4) # 进行非极大值抑制
return pred
```
最后,你可以在主函数中调用上述函数来进行目标检测并显示结果:
```python
def main():
image_file = 'path/to/your/image.jpg'
model_file = 'path/to/your/model.pt'
model, device = load_model(model_file)
image = Image.open(image_file).convert('RGB')
pred = detect_objects(image, model, device)
for det in pred[0]:
bbox = det[:4]
label = int(det[5])
score = det[4]
bbox = scale_coords(image.size, bbox) # 缩放边界框坐标
display(image.crop(bbox), label=label, score=score) # 显示目标图像及其标签和得分
if __name__ == '__main__':
main()
```
需要注意的是,以上代码只是一个简单示例。要运行YOLOv5,你需要准备好模型文件和图像文件,并相应地进行指定。此外,你还可以对代码进行扩展,以满足更具体的需求,比如使用摄像头实时检测等。
阅读全文
相关推荐
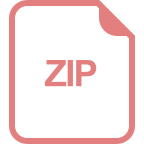
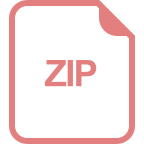
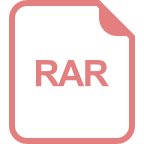















