不要使用第三方库
时间: 2023-07-11 08:15:35 浏览: 53
如果不使用第三方库,可以使用 C++ 标准库中的 `fstream` 和 `sstream` 来处理 Excel 文件和时间字符串,以及使用自定义排序函数来对时间字符串进行排序。
以下是读取 Excel 文件中第 11 行和第 12 行数据的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
#include <string>
int main() {
std::ifstream file("test.csv");
if (file.is_open()) {
std::string line;
std::vector<std::vector<std::string>> data;
int row = 0;
while (std::getline(file, line)) {
std::stringstream ss(line);
std::string cell;
std::vector<std::string> rowData;
int col = 0;
while (std::getline(ss, cell, ',')) {
if (row == 10 || row == 11) {
rowData.push_back(cell);
}
col++;
}
if (row == 10 || row == 11) {
data.push_back(rowData);
}
row++;
}
file.close();
for (const auto& rowData : data) {
for (const auto& cell : rowData) {
std::cout << cell << "\t";
}
std::cout << std::endl;
}
}
return 0;
}
```
该代码使用 `std::ifstream` 打开 Excel 文件,并使用 `std::getline` 函数逐行读取数据。通过判断行数和列数,筛选出第 11 行和第 12 行数据,并使用 `std::vector` 存储数据。最后输出第 11 行和第 12 行数据。
关于时间格式的排序,可以自定义排序函数,将时间字符串转换为时间戳(Unix 时间),然后按照时间戳排序。以下是一个简单的示例代码:
```c++
#include <iostream>
#include <vector>
#include <string>
#include <ctime>
#include <algorithm>
// 将时间字符串转换为 Unix 时间戳
time_t toTimestamp(const std::string& str) {
struct tm t;
time_t timestamp;
strptime(str.c_str(), "%Y-%m-%d %H:%M:%S", &t);
timestamp = mktime(&t);
return timestamp;
}
// 自定义排序函数
bool timeSort(const std::string& str1, const std::string& str2) {
time_t ts1 = toTimestamp(str1);
time_t ts2 = toTimestamp(str2);
return ts1 < ts2;
}
int main() {
std::vector<std::string> data = {"2021-10-01 10:30:00", "2021-10-02 09:00:00", "2021-10-01 08:00:00", "2021-10-03 12:00:00"};
std::sort(data.begin(), data.end(), timeSort);
for (const auto& str : data) {
std::cout << str << std::endl;
}
return 0;
}
```
该代码将时间字符串转换为 Unix 时间戳,并使用自定义排序函数 `timeSort` 对时间字符串进行排序,并输出排序后的结果。
相关推荐
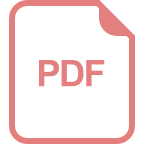
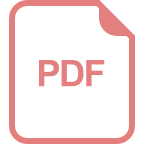
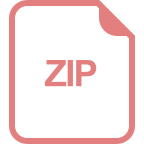














