stm32F103串口1基于库函数向串口调试工具打印字符串“soc:54% vol;3825 current;-2247 temp;315”例程
时间: 2024-02-01 15:16:14 浏览: 139
以下是基于库函数向串口1打印字符串的例程:
```c
#include "stm32f10x.h"
#include <stdio.h>
void USART1_Init(void);
void USART1_SendChar(char ch);
void USART1_SendString(char* str);
int main(void)
{
USART1_Init();
char buffer[50];
float voltage = 54.0;
int current = 3825;
int temp = -2247;
sprintf(buffer, "soc:%.0f%% vol;%d current;%d temp;%d\r\n", voltage, current, temp);
USART1_SendString(buffer);
while(1);
}
void USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// Enable clock for GPIOA and USART1
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
// Configure USART1 TX pin
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// Configure USART1 RX pin
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// Configure USART1
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// Enable USART1
USART_Cmd(USART1, ENABLE);
}
void USART1_SendChar(char ch)
{
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
USART_SendData(USART1, ch);
}
void USART1_SendString(char* str)
{
while(*str)
{
USART1_SendChar(*str++);
}
}
```
在以上例程中,我们使用了 `sprintf()` 函数将需要打印的字符串格式化到 `buffer` 数组中,然后使用 `USART1_SendString()` 函数向串口1发送该字符串。需要注意的是,在使用 `sprintf()` 函数时,需要将浮点数 `voltage` 的小数部分保留0位,因此格式字符串中使用了 `%.0f`。另外,为了保证字符串的完整性,我们在字符串末尾添加了回车换行符 `\r\n`。
在使用该例程时,需要将串口调试工具的波特率设置为115200,并连接到STM32F103的USART1的TX和GND引脚。
阅读全文
相关推荐
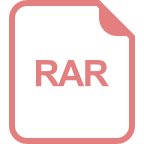
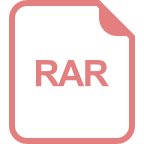
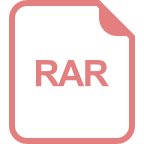
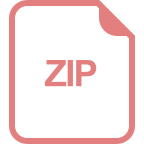
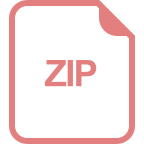
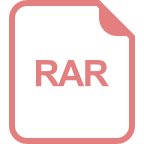
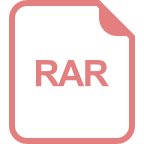
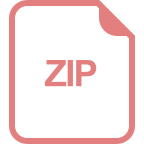
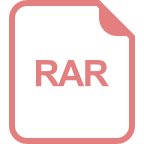
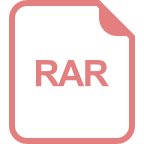
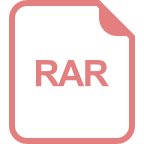
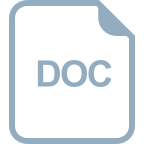
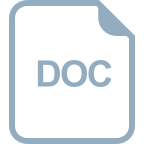
