贪心解决背包问题c++语言
时间: 2023-09-16 18:13:27 浏览: 98
好的,我可以回答关于贪心解决背包问题的问题。背包问题是一个经典的组合优化问题,贪心算法可以用于求解该问题。在贪心算法中,我们优先选择价值最高的物品放入背包,直到背包装满或没有物品可选。这种贪心策略可以得到较优的结果,但并不一定是最优解。在实际应用中,还需要结合动态规划等算法来解决更为复杂的背包问题。
相关问题
贪心算法背包问题c++
贪心算法是一种解决问题的策略,它在每一步选择中都采取在当前状态下最优(即看起来最好)的选择,希望这样的局部最优决策最终能够导致全局最优解。在背包问题中,特别是0-1背包问题,贪心算法常常用于求解物品装入容量有限的背包的最优化方案。
在C++中,经典的贪心算法解决背包问题通常是基于价值密度(单位重量的价值)来进行判断。例如,我们可以创建一个数组或vector存储每个物品的价值与其重量,然后按照价值密度从高到低排序。接下来,依次将每个物品添加到背包中,直到达到背包的容量。如果当前物品完全放入后剩余容量,那么就继续下一个物品;如果无法完全放入,则仅添加部分物品以最大化总价值。
这是一个简单的伪代码示例:
```cpp
bool canFit(int weight, int capacity) {
// 检查当前物品是否能完全放入背包
return weight <= capacity;
}
int greedyKnapsack(vector<pair<int, int>>& items, int capacity) {
sort(items.begin(), items.end(), [](const pair<int, int>& a, const pair<int, int>& b) { return (double)a.first / a.second > (double)b.first / b.second; }); // 按照价值密度降序排列
int totalValue = 0;
for (const auto& item : items) {
if (canFit(item.second, capacity)) {
totalValue += item.first;
capacity -= item.second; // 更新背包容量
} else {
totalValue += item.first * (capacity / item.second); // 如果不能全部放,取部分价值
break;
}
}
return totalValue;
}
```
请注意,这个贪心算法并不保证一定能得到背包问题的最优解,尤其是当存在相互依赖的物品时。
贪心法背包问题C++
以下是使用贪心法解决背包问题的C++代码示例:
```cpp
#include <iostream>
#include <algorithm>
using namespace std;
void Sort(int n, float v[], float w[]) {
// 根据单位重量内物品价值进行排序
float ratio[n];
for (int i = 0; i < n; i++) {
ratio[i] = v[i] / w[i];
}
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
if (ratio[j] < ratio[j+1]) {
swap(ratio[j], ratio[j+1]);
swap(v[j], v[j+1]);
swap(w[j], w[j+1]);
}
}
}
}
void Knapsack(int n, float M, float v[], float w[], float x[]) {
Sort(n, v, w);
for (int i = 0; i < n; i++) {
x[i] = 0;
}
float c = M;
for (int i = 0; i < n; i++) {
if (w[i] > c) {
break;
}
x[i] = 1;
c -= w[i];
}
if (i < n) {
x[i] = c / w[i];
}
}
int main() {
int n = 5; // 物品数
float M = 10; // 背包大小
float v[] = {60, 100, 120, 80, 70}; // 物品价值数组
float w[] = {10, 20, 30, 40, 50}; // 物品重量数组
float x[n]; // 占比数组
Knapsack(n, M, v, w, x);
cout << "物品占比:";
for (int i = 0; i < n; i++) {
cout << x[i] << " ";
}
cout << endl;
return 0;
}
```
阅读全文
相关推荐
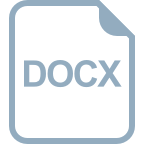

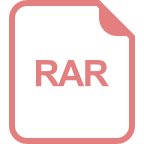










