c++ AES/ECB/PKCS5PADDING
时间: 2023-10-13 12:08:08 浏览: 165
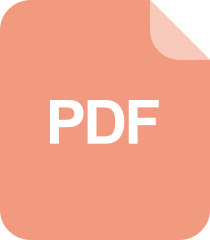
JAVA、Python、PHP一致的 "任意长度secret秘钥" 加解密AES/ECB/PKCS5Padding算法实现代码
您可以使用 OpenSSL 库来实现 C++ 中的 AES/ECB/PKCS5PADDING 加密。以下是一个简单的示例代码:
```c++
#include <openssl/aes.h>
#include <cstring>
void encrypt(unsigned char *plaintext, int plaintext_len, unsigned char *key,
unsigned char *iv, unsigned char *ciphertext) {
AES_KEY aes_key;
AES_set_encrypt_key(key, 128, &aes_key);
AES_encrypt(plaintext, ciphertext, &aes_key);
}
void decrypt(unsigned char *ciphertext, int ciphertext_len, unsigned char *key,
unsigned char *iv, unsigned char *plaintext) {
AES_KEY aes_key;
AES_set_decrypt_key(key, 128, &aes_key);
AES_decrypt(ciphertext, plaintext, &aes_key);
}
int main() {
unsigned char key[] = "0123456789abcdef";
unsigned char iv[] = "0123456789abcdef";
unsigned char plaintext[] = "Hello, world!";
int plaintext_len = strlen((char *)plaintext);
// 加密
unsigned char ciphertext[plaintext_len];
encrypt(plaintext, plaintext_len, key, iv, ciphertext);
// 解密
unsigned char decryptedtext[plaintext_len];
decrypt(ciphertext, plaintext_len, key, iv, decryptedtext);
// 输出结果
printf("原文: %s\n", plaintext);
printf("密文: ");
for (int i = 0; i < plaintext_len; i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
printf("解密后: %s\n", decryptedtext);
return 0;
}
```
阅读全文
相关推荐
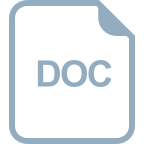
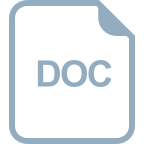
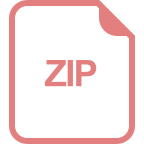
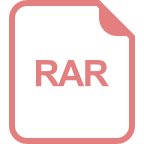
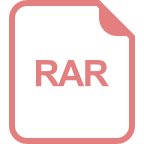
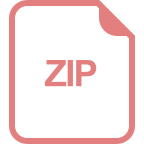
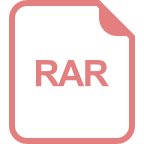
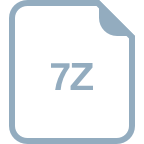
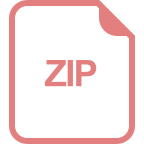
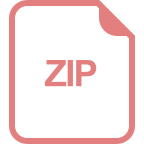
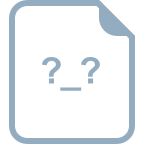
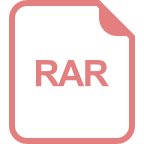
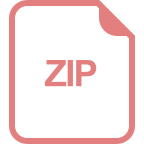
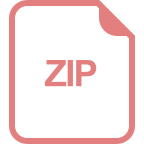


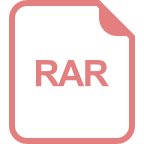