C++如何发送post请求
时间: 2024-09-09 09:00:51 浏览: 53
在C++中,由于标准库并不直接支持HTTP请求,通常我们会使用一些第三方库如cURL(libcurl)来发送POST请求。以下是一个简单的示例,展示如何使用cURL发送POST数据:
```cpp
#include <curl/curl.h>
size_t writeCallback(char* ptr, size_t size, size_t nmemb, std::string* data) {
data->append(ptr, size * nmemb);
return size * nmemb;
}
void sendPostRequest(const std::string& url, const std::string& postData) {
CURL* curl = curl_easy_init();
if (curl) {
std::string buffer;
curl_easy_setopt(curl, CURLOPT_URL, url.c_str());
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, writeCallback);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &buffer);
// 设置POST请求头,告诉服务器我们要发送的数据类型(通常是application/x-www-form-urlencoded)
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, {"Content-Type: application/x-www-form-urlencoded"});
curl_easy_setopt(curl, CURLOPT_POST, 1L);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, postData.c_str());
// 执行请求并处理结果
CURLcode res = curl_easy_perform(curl);
if (res != CURLE_OK) {
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
} else {
std::cout << "Response: " << buffer << std::endl;
}
// 清理资源
curl_easy_cleanup(curl);
}
}
```
在这个例子中,你需要链接cURL库,并确保已经包含了`<curl/curl.h>`头文件。使用`sendPostRequest`函数时,传入你要访问的URL和POST数据。
阅读全文
相关推荐
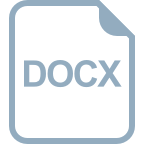
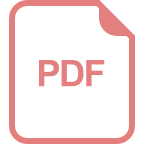
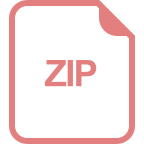

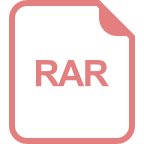













