c++ c++ 实现 发送http get/post请求 简单版本
时间: 2023-07-03 07:02:14 浏览: 177
### 回答1:
C语言标准库中没有提供直接发送HTTP请求的函数,但是可以使用一些第三方库来实现发送HTTP GET/POST请求的功能,比如libcurl。
libcurl是一个非常强大的开源网络传输库,提供了丰富的功能和易于使用的API。下面是一个简单版本的使用libcurl库发送HTTP GET/POST请求的示例:
1. 导入头文件和初始化libcurl:
#include <stdio.h>
#include <curl/curl.h>
int main(void) {
CURL *curl;
CURLcode res;
curl_global_init(CURL_GLOBAL_DEFAULT);
curl = curl_easy_init();
2. 设置请求的URL:
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
3. 发送HTTP GET请求:
res = curl_easy_perform(curl);
4. 发送HTTP POST请求:
if(res != CURLE_OK)
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
else {
// 设置POST请求参数
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "param1=value1¶m2=value2");
// 发送POST请求
res = curl_easy_perform(curl);
}
5. 检查请求是否成功:
if(res != CURLE_OK)
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
6. 清理和释放资源:
curl_easy_cleanup(curl);
}
curl_global_cleanup();
return 0;
}
以上是一个简单的使用libcurl库发送HTTP GET/POST请求的示例代码。你可以根据自己的需要进行修改和扩展。
### 回答2:
在C语言中,我们可以使用libcurl库来实现发送HTTP GET/POST请求的简单版本。
首先,我们需要安装并引入libcurl库。在Ubuntu系统中,我们可以使用以下命令安装libcurl库:
```
sudo apt-get install libcurl4-openssl-dev
```
然后,我们可以创建一个C源文件,并包含`curl/curl.h`头文件:
```c
#include <stdio.h>
#include <curl/curl.h>
```
接下来,我们可以定义一个函数来发送HTTP GET请求,并使用libcurl库完成请求的发送和接收:
```c
void sendHttpGetRequest(const char* url) {
CURL *curl;
CURLcode res;
// 初始化libcurl
curl_global_init(CURL_GLOBAL_DEFAULT);
// 创建一个curl句柄
curl = curl_easy_init();
if(curl) {
// 设置要请求的URL
curl_easy_setopt(curl, CURLOPT_URL, url);
// 执行GET请求
res = curl_easy_perform(curl);
// 检查请求是否成功
if(res != CURLE_OK)
fprintf(stderr, "curl_easy_perform() failed: %s\n",
curl_easy_strerror(res));
// 清理curl句柄
curl_easy_cleanup(curl);
}
// 清理libcurl
curl_global_cleanup();
}
```
同样地,我们也可以定义一个函数来发送HTTP POST请求,只需要对发送的数据进行适当的设置即可:
```c
void sendHttpPostRequest(const char* url, const char* postData) {
CURL *curl;
CURLcode res;
// 初始化libcurl
curl_global_init(CURL_GLOBAL_DEFAULT);
// 创建一个curl句柄
curl = curl_easy_init();
if(curl) {
// 设置要请求的URL
curl_easy_setopt(curl, CURLOPT_URL, url);
// 设置POST请求的数据
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, postData);
// 执行POST请求
res = curl_easy_perform(curl);
// 检查请求是否成功
if(res != CURLE_OK)
fprintf(stderr, "curl_easy_perform() failed: %s\n",
curl_easy_strerror(res));
// 清理curl句柄
curl_easy_cleanup(curl);
}
// 清理libcurl
curl_global_cleanup();
}
```
以上代码实现了一个简单版本的发送HTTP GET/POST请求的功能。在发送请求之前,我们需要设置要请求的URL,并可以选择设置POST请求的数据。发送请求之后,我们还可以对返回结果进行适当的处理,根据需要进行错误处理。
当然,这只是一个简单的示例代码。在实际应用中,可能还需要处理更多的HTTP请求选项和参数,以满足具体的需求。
### 回答3:
C语言本身不直接提供发送HTTP请求的功能,但可以通过使用第三方库来实现发送HTTP GET/POST请求的功能。下面给出一个使用libcurl库(一个广泛使用的C库,用于支持各种协议的网络操作)的简单版本示例:
```c
#include <stdio.h>
#include <curl/curl.h>
int main() {
CURL *curl;
CURLcode res;
curl_global_init(CURL_GLOBAL_ALL); // 初始化libcurl
curl = curl_easy_init(); // 初始化一个CURL对象
if(curl) {
char *url = "http://example.com"; // 请求的URL
curl_easy_setopt(curl, CURLOPT_URL, url); // 设置要请求的URL
// 执行GET请求
res = curl_easy_perform(curl);
if(res != CURLE_OK) {
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
}
curl_easy_cleanup(curl); // 清理curl对象
}
curl_global_cleanup(); // 清理libcurl
return 0;
}
```
上述代码使用了libcurl库的`curl_easy_init()`和`curl_easy_setopt()`函数设置请求的URL,并使用`curl_easy_perform()`函数执行GET请求。你可以通过修改`char *url`的值为你要请求的具体URL来发送GET请求。
如果要发送POST请求,你还需要添加一些额外的代码来设置请求体和请求头,以及使用`curl_easy_setopt()`函数设置请求方法为POST。这里提供一个示例来发送带有请求体的POST请求:
```c
#include <stdio.h>
#include <curl/curl.h>
int main() {
CURL *curl;
CURLcode res;
curl_global_init(CURL_GLOBAL_ALL);
curl = curl_easy_init();
if(curl) {
char *url = "http://example.com";
char *data = "param1=value1¶m2=value2"; // POST请求的请求体
curl_easy_setopt(curl, CURLOPT_URL, url);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, data); // 设置请求体
// 设置请求头
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "Content-Type: application/x-www-form-urlencoded");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
// 设置请求方法为POST
curl_easy_setopt(curl, CURLOPT_POST, 1L);
res = curl_easy_perform(curl);
if(res != CURLE_OK) {
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
}
curl_slist_free_all(headers); // 释放请求头的内存
curl_easy_cleanup(curl);
}
curl_global_cleanup();
return 0;
}
```
上述代码在GET请求的基础上,增加了设置请求体和请求头的代码,以及设置`CURLOPT_POST`选项为`1L`来指定POST方法。你可以根据自己的需求修改`char *url`和`char *data`的值来发送不同的POST请求。
相关推荐
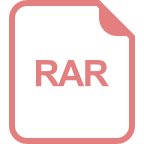














