verlog输出正弦波
时间: 2024-09-23 19:08:14 浏览: 30
Verilog是一种硬件描述语言(HDL),主要用于设计和验证数字电路的行为。要在Verilog中输出正弦波信号,通常需要结合模拟部分和触发器来实现。以下是一个简单的示例,展示如何使用Verilog生成一个基本的方波,而不是精确的正弦波,因为直接生成无限精度正弦波在硬件上比较复杂:
```verilog
module sine_generator(
input wire clk, // 时钟输入
output reg [7:0] out // 输出8位正弦波模拟值
);
reg [7:0] counter; // 计数器用于周期性变化
always @(posedge clk) begin
if (counter == 255) begin
counter <= 0; // 当计数满时,重置计数
} else begin
counter <= counter + 1; // 否则增加计数
out = sin(counter / 256 * 2 * $pi); // 用计数值计算近似的正弦值
end
end
// 其他必要的库函数和仿真配置
endmodule
```
在这个例子中,`sin`函数通常是假设的,实际上Verilog核心并不内置这种数学函数,需要依赖特定的数学库支持或者外部工具如ModelSim等进行仿真。而且,由于硬件限制,实际的正弦波生成通常是通过数字滤波器或专用的模拟IP块完成。
相关问题
Veirlog HDL正弦波信号发生器功能。正弦波信号幅度可调设计结构部分
Verilog HDL 正弦波信号发生器可以使用 DDS(直接数字合成)技术来实现。DDS 技术使用一个参考时钟信号和一个可编程的相位累加器来产生一个数字控制的正弦波输出。下面是一个简单的 Verilog HDL 正弦波信号发生器的实现:
```verilog
module sine_generator(
input clk, // 参考时钟信号
input [7:0] amplitude, // 正弦波幅度
output reg signed [15:0] sine // 正弦波输出
);
reg signed [15:0] phase_accumulator; // 相位累加器
always @(posedge clk) begin
phase_accumulator <= phase_accumulator + 10'b0110101010; // 每个时钟周期相位增加一个固定步进值,控制正弦波的频率
sine <= amplitude * $sin(phase_accumulator[15:6]); // $sin 函数返回相位所对应的正弦值,乘以幅度即可得到正弦波信号输出
end
endmodule
```
在这个例子中,我们使用了一个 16 位有符号相位累加器和一个 8 位幅度输入信号。使用一个固定的步进值控制相位累加器的增加,从而控制正弦波的频率。$sin$ 函数使用相位值计算正弦波值,并乘以幅度输入得到最终的正弦波输出。
要实现可调节幅度的正弦波信号发生器,可以在输入端添加一个幅度输入信号,用它来控制正弦波的幅度。在上面的例子中,我们将幅度输入视为 8 位无符号整数,但是如果需要更高的精度,可以增加幅度输入的位数。
verilog HDL输出三角波 正弦波 方波 脉冲波
下面是 Verilog HDL 实现输出三角波、正弦波、方波和脉冲波的例子:
三角波:
```
module triangle_wave(
input clk,
input rst,
output reg out
);
reg [7:0] count;
initial begin
count <= 0;
out <= 0;
end
always @(posedge clk or posedge rst) begin
if (rst) begin
count <= 0;
out <= 0;
end else begin
if (count == 8'hFF) begin
count <= 0;
end else begin
count <= count + 1;
end
if (count <= 8'h7F) begin
out <= count;
end else begin
out <= ~count + 1;
end
end
end
endmodule
```
正弦波:
```
module sine_wave(
input clk,
input rst,
output reg signed [7:0] out
);
reg [7:0] count;
initial begin
count <= 0;
out <= 0;
end
always @(posedge clk or posedge rst) begin
if (rst) begin
count <= 0;
out <= 0;
end else begin
count <= count + 1;
out <= $signed(64.0 + 64.0 * $sin(count * 2.0 * $pi / 256.0));
end
end
endmodule
```
方波:
```
module square_wave(
input clk,
input rst,
output reg out
);
reg [7:0] count;
initial begin
count <= 0;
out <= 0;
end
always @(posedge clk or posedge rst) begin
if (rst) begin
count <= 0;
out <= 0;
end else begin
if (count == 8'hFF) begin
count <= 0;
out <= ~out;
end else begin
count <= count + 1;
end
end
end
endmodule
```
脉冲波:
```
module pulse_wave(
input clk,
input rst,
output reg out
);
reg [7:0] count;
initial begin
count <= 0;
out <= 0;
end
always @(posedge clk or posedge rst) begin
if (rst) begin
count <= 0;
out <= 0;
end else begin
if (count == 8'h7F) begin
count <= 0;
out <= 1;
end else begin
count <= count + 1;
out <= 0;
end
end
end
endmodule
```
这些例子中,计数器的位宽和计数的范围可以根据需要进行调整。同时,三角波和正弦波的输出信号使用了有符号的变量,需要使用 `$signed` 函数进行转换。
阅读全文
相关推荐
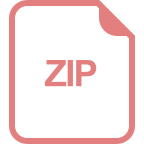
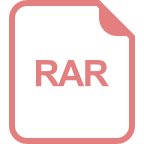
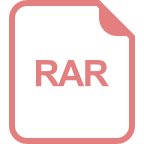
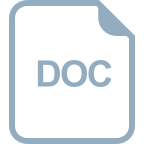
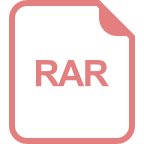
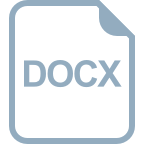





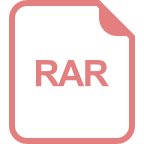
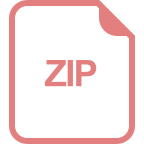
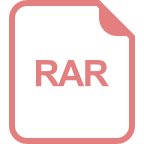
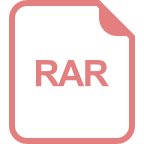
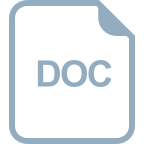
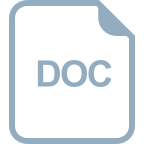