devc++用后缀表达式求值
时间: 2023-11-24 14:52:12 浏览: 104
在DevC++中使用后缀表达式求值的方法如下:
1. 首先,你需要定义一个栈来存储操作数。
2. 遍历后缀表达式中的每个字符。
3. 如果遇到操作数,将其压入栈中。
4. 如果遇到操作符,从栈中弹出两个操作数,并根据操作符进行计算,将计算结果压入栈中。
5. 继续遍历表达式,直到遍历完所有字符。
6. 最后,栈中剩下的唯一元素就是后缀表达式的计算结果。
下面是一个示例代码,演示了如何在DevC++中使用后缀表达式求值:
```cpp
#include <iostream>
#include <stack>
#include <string>
#include <cctype>
using namespace std;
int evaluatePostfix(string postfix) {
stack<int> operands;
for (char c : postfix) {
if (isdigit(c)) {
operands.push(c - '0');
} else if (c == '+' || c == '-' || c == '*' || c == '/') {
int operand2 = operands.top();
operands.pop();
int operand1 = operands.top();
operands.pop();
switch (c) {
case '+':
operands.push(operand1 + operand2);
break;
case '-':
operands.push(operand1 - operand2);
break;
case '*':
operands.push(operand1 * operand2);
break;
case '/':
operands.push(operand1 / operand2);
break;
}
}
}
return operands.top();
}
int main() {
string postfix = "52+3*";
int result = evaluatePostfix(postfix);
cout << "Result: " << result << endl;
return 0;
}
```
这段代码演示了如何使用后缀表达式 "52+3*" 求值,结果为 21。
阅读全文
相关推荐
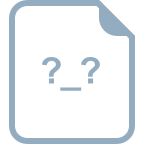


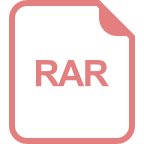
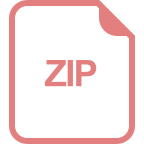
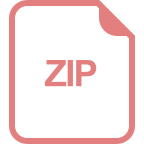
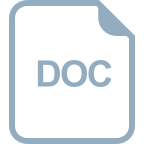


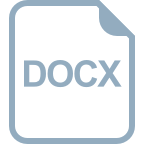
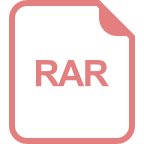
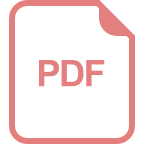
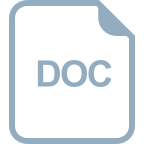