1.读取指定离线鸢尾花数据集,按照要求完成如下任务. 1.1建立Logistic的3阶多项式; 1.2 训练前两个特征,并输出准确率; 1.3绘制最终分类图;
时间: 2024-10-19 18:02:39 浏览: 17
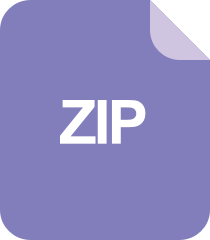
logistic回归分类器鸢尾花_logistic回归_logistic回归分类器_回归分析_鸢尾花数据集_

首先,你需要准备一个鸢尾花数据集,通常这类数据集可以在Python的scikit-learn库中找到,如`iris`数据集就是一个经典的例子。为了完成这个任务,你可以遵循以下步骤:
1. **导入所需的库**:
```python
import numpy as np
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.preprocessing import PolynomialFeatures
from sklearn.metrics import accuracy_score
import matplotlib.pyplot as plt
```
2. **加载并预处理数据**:
- 加载鸢尾花数据
```python
iris = load_iris()
X = iris.data[:, :2] # 取前两个特征
y = iris.target
```
- 将类别标签转换为数值形式,以便于模型训练
```python
y_encoded = np.argmax(y.reshape(-1, 1), axis=1)
```
3. **构建3阶多项式特征**:
```python
poly = PolynomialFeatures(degree=3)
X_poly = poly.fit_transform(X)
```
4. **分割数据集(训练集和测试集)**:
```python
X_train, X_test, y_train, y_test = train_test_split(X_poly, y_encoded, test_size=0.2, random_state=42)
```
5. **训练Logistic回归模型**:
```python
model = LogisticRegression(solver='lbfgs') # 使用L-BFGS优化器
model.fit(X_train, y_train)
```
6. **评估模型**:
- 预测测试集结果
```python
y_pred = model.predict(X_test)
```
- 计算前两个特征的准确率
```python
accuracy = accuracy_score(y_test, y_pred)
print(f"前两个特征的准确率为: {accuracy * 100:.2f}%")
```
7. **绘制最终分类图**:
- 可能需要一些额外的库(比如`seaborn`或`plotly`),这里展示基础版本
```python
plt.figure(figsize=(8, 6))
plt.scatter(X_test[:, 0], X_test[:, 1], c=y_pred, cmap='viridis')
decision_boundary = model.coef_[0][0] * X_test[:, 0] + model.coef_[0][1] * X_test[:, 1]
plt.plot(X_test[:, 0], decision_boundary, 'k-', lw=2)
plt.xlabel('Feature 1')
plt.ylabel('Feature 2')
plt.title("Final Classification Plot with Logistic Regression (Polynomial)")
plt.show()
阅读全文
相关推荐
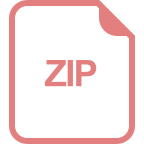
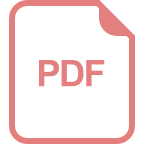








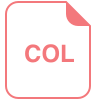
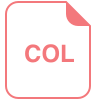
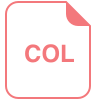
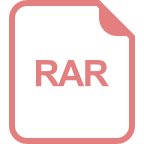
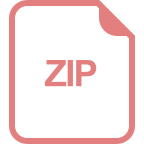
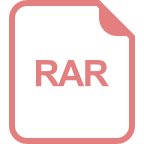
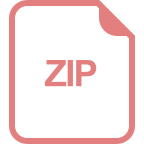