shell排序c++
时间: 2023-11-18 18:51:28 浏览: 59
shell排序是一种基于插入排序的排序算法。它通过将待排序的元素按照一定的间隔分组,对每组进行插入排序,然后逐渐减小间隔,直到间隔为1,完成最后一次插入排序,从而使整个序列基本有序。
在C语言中,可以使用以下代码实现shell排序:
```c++
void shell(int *arr, int len) {
for (int gap = len / 2; gap > 0; gap /= 2) {
for (int i = gap; i < len; i++) {
int temp = arr[i];
int j;
for (j = i; j >= gap && arr[j - gap] > temp; j -= gap) {
arr[j] = arr[j - gap];
}
arr[j] = temp;
}
}
}
```
相关问题
shell排序算法c++
当你说到“shell排序算法”时,我想你可能指的是“希尔排序”算法,这是一种基于插入排序的排序算法,可以在较短的时间内对相对较大的数据集进行排序。
以下是使用C++实现的希尔排序算法的代码示例:
```c++
#include <iostream>
using namespace std;
void shellSort(int arr[], int n) {
for (int gap = n / 2; gap > 0; gap /= 2) {
for (int i = gap; i < n; i += 1) {
int temp = arr[i];
int j;
for (j = i; j >= gap && arr[j - gap] > temp; j -= gap) {
arr[j] = arr[j - gap];
}
arr[j] = temp;
}
}
}
int main() {
int arr[] = {12, 34, 54, 2, 3};
int n = sizeof(arr)/sizeof(arr[0]);
cout << "Original array: ";
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
cout << endl;
shellSort(arr, n);
cout << "Sorted array: ";
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
cout << endl;
return 0;
}
```
这段代码中,`shellSort`函数实现了希尔排序算法。它通过循环遍历不同间隔(gap)的子序列来排序,初始间隔值通常设置为n/2,并在每个迭代中将间隔值减半。在每个间隔内,算法使用插入排序对子序列进行排序。
在`main`函数中,我们定义了一个整数数组并对其进行排序。在输出原始数组和排序后的数组之后,程序返回0,表示成功运行。
希尔排序c++代码
希尔排序(Shell Sort)是插入排序的一种改进版本,通过将待排序数据划分为若干个子序列分别进行插入排序,从而达到排序的目的。希尔排序的时间复杂度为O(nlogn),相较于插入排序的O(n^2)具有更好的性能。
以下是一个基于C++的希尔排序代码示例:
```c++
#include <iostream>
#include <vector>
void shellSort(std::vector<int>& nums) {
int n = nums.size();
for(int gap = n / 2; gap > 0; gap /= 2) {
for(int i = gap; i < n; i++) {
int temp = nums[i];
int j;
for(j = i; j >= gap && nums[j - gap] > temp; j -= gap) {
nums[j] = nums[j - gap];
}
nums[j] = temp;
}
}
}
int main() {
std::vector<int> nums = {5, 2, 6, 0, 3, 9, 1, 7, 4, 8};
shellSort(nums);
for(int num : nums) {
std::cout << num << " ";
}
std::cout << std::endl;
return 0;
}
```
在上述代码中,我们定义了一个名为`shellSort()`的函数,用于对输入的vector进行希尔排序。首先,我们获取输入vector的长度n,并设置一个初始的间隔gap为n/2。随后,我们在一个循环中不断将gap折半,直到gap为0结束循环。在每次循环中,我们从第gap个元素开始遍历整个vector,对每个元素进行插入排序操作。具体来说,我们将当前元素保存到temp中,然后从当前元素往前遍历,如果前面的元素大于temp,则将前面的元素后移gap个位置,直到找到一个比temp小的元素,然后将temp插入到该元素的后面gap个位置处。最后,我们使用一个循环输出已排序的vector。
需要注意的是,在实际应用中,我们可能需要根据具体数据的特点选择不同的间隔序列,以达到更好的效果。
相关推荐
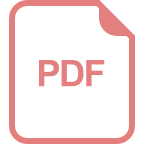
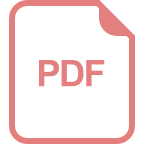












