用python实验2. 设计三维向量类】设计一个三维向量类,并实现向量的加法、减法以及向量与标量的乘法 和除法运算
时间: 2024-11-27 08:18:50 浏览: 20
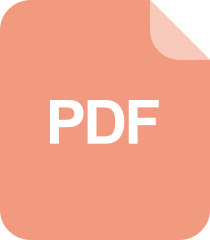
纹理坐标-python实现pdf转换成word/txt纯文本文件
在Python中,我们可以创建一个名为`Vector3D`的类,来表示三维向量并实现基本的数学操作。这里是一个简单的实现:
```python
class Vector3D:
def __init__(self, x=0, y=0, z=0):
self.x = x
self.y = y
self.z = z
# 向量加法
def __add__(self, other):
if isinstance(other, Vector3D):
return Vector3D(self.x + other.x, self.y + other.y, self.z + other.z)
else:
raise ValueError("Cannot add a vector to a non-vector")
# 向量减法
def __sub__(self, other):
if isinstance(other, Vector3D):
return Vector3D(self.x - other.x, self.y - other.y, self.z - other.z)
else:
raise ValueError("Cannot subtract a vector from a non-vector")
# 向量与标量乘法(点积)
def __mul__(self, scalar):
if isinstance(scalar, (int, float)):
return Vector3D(self.x * scalar, self.y * scalar, self.z * scalar)
else:
raise ValueError("Cannot multiply a vector by a non-scalar")
# 向量与标量除法(相当于缩小向量长度)
def __truediv__(self, scalar):
if isinstance(scalar, (int, float)) and scalar != 0:
magnitude = scalar
else:
raise ValueError("Cannot divide a vector by zero")
return Vector3D(self.x / magnitude, self.y / magnitude, self.z / magnitude)
# 示例
v1 = Vector3D(1, 2, 3)
v2 = Vector3D(4, 5, 6)
print(f"v1: {v1}, v2: {v2}")
# 加法
result_add = v1 + v2
print(f"v1 + v2: {result_add}")
# 减法
result_sub = v1 - v2
print(f"v1 - v2: {result_sub}")
# 与标量相乘
product_scalar = v1 * 2
print(f"v1 * 2: {product_scalar}")
# 与标量相除
normalized_v1 = v1 / 5
print(f"v1 / 5 (normalized): {normalized_v1}")
```
阅读全文
相关推荐
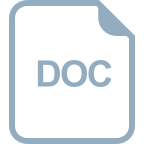
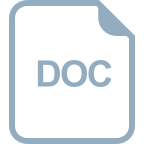















