使用python语言,设计一个三维向量类,并实现向量的加法、减法以及向量与标量的乘法和除法运算。
时间: 2024-11-25 07:32:10 浏览: 26
在Python中,我们可以设计一个名为`Vector3D`的三维向量类,包括属性`x`, `y`, 和 `z`,以及用于加法、减法、标量乘法和标量除法的方法。下面是一个简单的实现示例:
```python
class Vector3D:
def __init__(self, x=0, y=0, z=0):
self.x = x
self.y = y
self.z = z
# 向量加法
def add(self, other):
if isinstance(other, Vector3D):
return Vector3D(self.x + other.x, self.y + other.y, self.z + other.z)
else:
raise ValueError("只能对另一个Vector3D对象执行加法")
# 向量减法
def subtract(self, other):
if isinstance(other, Vector3D):
return Vector3D(self.x - other.x, self.y - other.y, self.z - other.z)
else:
raise ValueError("只能对另一个Vector3D对象执行减法")
# 标量乘法
def scale(self, scalar):
if isinstance(scalar, (int, float)):
return Vector3D(self.x * scalar, self.y * scalar, self.z * scalar)
else:
raise ValueError("只能对标量数值执行乘法")
# 标量除法(如果除数非零)
def divide(self, scalar):
if isinstance(scalar, (int, float)) and scalar != 0:
return Vector3D(self.x / scalar, self.y / scalar, self.z / scalar)
else:
raise ValueError("除数不能为零")
# 示例用法
vector_a = Vector3D(1, 2, 3)
vector_b = Vector3D(4, 5, 6)
vector_sum = vector_a.add(vector_b)
print(f"向量加法结果: {vector_sum.x}, {vector_sum.y}, {vector_sum.z}")
scalar_multiply = vector_a.scale(2)
print(f"标量乘法结果: {scalar_multiply.x}, {scalar_multiply.y}, {scalar_multiply.z}")
# 除法需注意避免除以零错误
try:
scalar_divide = vector_a.divide(2)
print(f"标量除法结果: {scalar_divide.x}, {scalar_divide.y}, {scalar_divide.z}")
except ValueError as e:
print(e)
```
这个`Vector3D`类实现了基本的向量操作,但在实际项目中可能需要添加更多的功能,如长度计算、单位化、点积等。
阅读全文
相关推荐
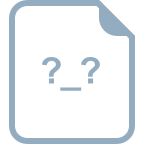
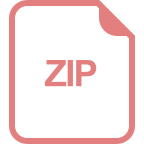
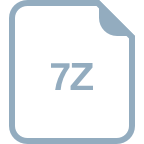















