c++中怎么测长度为零链表的长度
时间: 2024-10-28 13:11:49 浏览: 19
在 C++ 中,要计算一个空链表(即长度为零的链表)的长度,通常的做法是在遍历链表之前先检查头节点是否为空。如果头节点是 nullptr 或者 NULL,则表示链表为空,其长度为0。如果你有一个指针指向链表的头结点,你可以这样做:
```cpp
struct ListNode {
int val;
ListNode *next;
ListNode() : val(0), next(nullptr) {} // 默认构造函数
};
int getLinkedListLength(ListNode* head) {
if (head == nullptr) { // 检查链表是否为空
return 0; // 链表长度为0
} else {
int length = 1; // 初始化长度为1,因为已知有一个元素
ListNode* current = head;
while (current->next != nullptr) { // 遍历链表直到遇到尾节点
length++;
current = current->next;
}
return length; // 返回链表的实际长度
}
}
```
在这个例子中,`getLinkedListLength` 函数会返回链表的有效节点数。如果链表为空,它直接返回0。
相关问题
c++建立长度为n的链表
下面是一个简单的示例,展示如何使用 C++ 建立长度为 n 的链表:
```c++
#include <iostream>
using namespace std;
// 定义链表节点结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 建立长度为 n 的链表
ListNode* buildList(int n) {
if (n == 0) return NULL;
ListNode* head = new ListNode(0);
ListNode* cur = head;
for (int i = 1; i <= n; i++) {
ListNode* node = new ListNode(i);
cur->next = node;
cur = cur->next;
}
return head->next;
}
// 打印链表
void printList(ListNode* head) {
ListNode* cur = head;
while (cur) {
cout << cur->val << " ";
cur = cur->next;
}
cout << endl;
}
int main() {
ListNode* head = buildList(5);
printList(head);
return 0;
}
```
在上面的示例中,我们首先定义了一个 `ListNode` 结构体,用来表示链表节点,包含一个值域 `val` 和一个指向下一个节点的指针 `next`。
然后,我们定义了一个函数 `buildList`,用来建立长度为 n 的链表。在函数中,我们首先创建一个头节点 `head`,然后循环 n 次,创建 n 个节点,并将它们按顺序链接在一起。最后返回头节点的下一个节点,即整个链表的头节点。
最后,我们定义了一个函数 `printList`,用来打印链表。在函数中,我们从头节点开始遍历整个链表,依次输出每个节点的值域。
在 `main` 函数中,我们调用 `buildList` 函数建立了一个长度为 5 的链表,然后调用 `printList` 函数打印了这个链表。
c++中链表长度怎么求
在C++中求链表的长度可以通过遍历链表的方式来实现。你可以定义一个变量来计数链表中节点的数量,然后在遍历链表时,每遇到一个节点就将计数器加一。下面是一个示例代码:
```cpp
#include <iostream>
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
int getListLength(ListNode* head) {
int length = 0;
ListNode* current = head;
while (current != nullptr) {
length++;
current = current->next;
}
return length;
}
int main() {
// 创建链表
ListNode* head = new ListNode(1);
ListNode* node1 = new ListNode(2);
ListNode* node2 = new ListNode(3);
head->next = node1;
node1->next = node2;
// 求链表长度
int length = getListLength(head);
std::cout << "链表长度为:" << length << std::endl;
// 释放链表内存
delete node2;
delete node1;
delete head;
return 0;
}
```
在上面的示例代码中,我们定义了一个 `getListLength` 函数来计算链表的长度。通过不断更新 `current` 指针,直到指向最后一个节点的 `next` 为 `nullptr`,就可以统计出链表中节点的数量。最后在 `main` 函数中,我们创建了一个包含3个节点的链表,并输出了链表的长度为3。
阅读全文
相关推荐
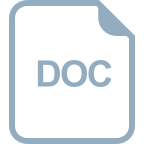
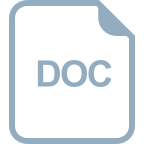
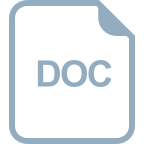

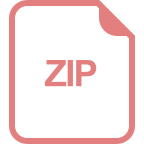











