RequestBody body=RequestBody.create(jsonBody.toString(),JSON);
时间: 2024-08-29 09:01:15 浏览: 27
这行代码是在Android或Spring Boot等Web开发框架中常见的,用于创建HTTP请求体(RequestBody)。`RequestBody.create()` 方法是一个工厂函数,用于将给定的数据转换为可以发送到服务器的请求体。
- `jsonBody.toString()`:这里假设`jsonBody`是一个包含了JSON数据的对象或者集合,`toString()`方法将其转化为String形式,因为`RequestBody`接受的是字符串或者其他支持序列化的类型。
- `JSON`:通常这是一个指定的MIME类型(Media Type Identifier),表示请求体内容的编码格式,比如 "application/json",表明数据是以JSON格式的。
整体上,这一句的意思是创建一个新的`RequestBody`实例,它的内容是从`jsonBody`对象转换得到的JSON字符串,并且设置了MIME类型为JSON。
相关问题
RequestBody body = RequestBody.create(JSON_TYPE, param); 报错
如果报错信息如下:
```
The method create(MediaType, String) in the type RequestBody is not applicable for the arguments (MediaType, JSONObject)
```
或
```
The method create(MediaType, String) in the type RequestBody is not applicable for the arguments (MediaType, Map<String, Object>)
```
这是因为 `RequestBody.create()` 方法的第二个参数需要是一个字符串类型的数据,而你传入的参数是一个 `JSONObject` 或 `Map<String, Object>` 类型的数据。
解决方法是将 `JSONObject` 或 `Map<String, Object>` 类型的数据转换成字符串类型。例如,你可以使用以下代码将 `JSONObject` 类型的数据转换成字符串类型:
```
JSONObject jsonObject = new JSONObject();
jsonObject.put("name", "John");
jsonObject.put("age", 30);
String jsonStr = jsonObject.toString();
RequestBody body = RequestBody.create(JSON_TYPE, jsonStr);
```
或者,你可以使用以下代码将 `Map<String, Object>` 类型的数据转换成字符串类型:
```
Map<String, Object> map = new HashMap<String, Object>();
map.put("name", "John");
map.put("age", 30);
String jsonStr = new Gson().toJson(map);
RequestBody body = RequestBody.create(JSON_TYPE, jsonStr);
```
其中,`Gson` 是 Google 提供的一个用于将 Java 对象转换成 JSON 格式的库。你需要在项目中添加 Gson 的依赖,例如:
```
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.5</version>
</dependency>
```
convertBtn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { String text2 = editText2.getText().toString(); String apiUrl = "https://yurl6.cn/user/api.php"; String curl = "curl=" + text2; OkHttpClient client = new OkHttpClient(); MediaType mediaType = MediaType.parse("application/json"); RequestBody requestBody = RequestBody.create(mediaType, "{\"curl\":\"" + curl + "\"}"); Request request = new Request.Builder() .url(apiUrl) .post(requestBody) .build(); try { Response response = client.newCall(request).execute(); String result = response.body().string(); JSONObject jsonObject = new JSONObject(result); String shortUrl = jsonObject.getString("shortUrl"); editText3.setText(shortUrl); } catch (IOException e) { e.printStackTrace(); } catch (JSONException e) { e.printStackTrace(); } } }); } 在点击按钮后,app闪退
闪退可能是由于一些异常情况引起的,例如网络连接异常或JSON解析错误等。你可以在捕获异常的代码块中打印出异常信息来调试代码。请尝试以下修改,看看是否能够解决问题:
```java
convertBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String text2 = editText2.getText().toString();
String apiUrl = "https://yurl6.cn/user/api.php";
String curl = "curl=" + text2;
OkHttpClient client = new OkHttpClient();
MediaType mediaType = MediaType.parse("application/json");
RequestBody requestBody = RequestBody.create(mediaType, "{\"curl\":\"" + curl + "\"}");
Request request = new Request.Builder()
.url(apiUrl)
.post(requestBody)
.build();
try {
Response response = client.newCall(request).execute();
String result = response.body().string();
JSONObject jsonObject = new JSONObject(result);
String shortUrl = jsonObject.getString("shortUrl");
editText3.setText(shortUrl);
} catch (IOException | JSONException e) {
e.printStackTrace();
}
}
});
```
同时,请确保你已经在Manifest.xml中声明了网络权限:
```xml
<uses-permission android:name="android.permission.INTERNET" />
```
相关推荐
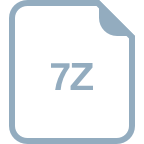
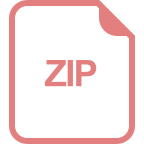
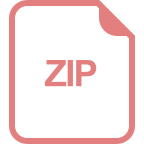












