用c语言实现贪心算法01背包问题
时间: 2024-03-15 09:40:10 浏览: 193
贪心算法是一种常用的解决最优化问题的方法,其中01背包问题是贪心算法的一个经典应用之一。在C语言中实现贪心算法解决01背包问题,可以按照以下步骤进行:
1. 首先,定义一个结构体来表示物品,包括物品的价值和重量。例如:
```c
struct Item {
int value;
int weight;
};
```
2. 接下来,定义一个函数来比较两个物品的单位价值(即价值与重量的比值),并按照单位价值从大到小排序物品数组。例如:
```c
int compare(const void* a, const void* b) {
struct Item* item1 = (struct Item*)a;
struct Item* item2 = (struct Item*)b;
double ratio1 = (double)item1->value / item1->weight;
double ratio2 = (double)item2->value / item2->weight;
if (ratio1 < ratio2) {
return 1;
} else if (ratio1 > ratio2) {
return -1;
} else {
return 0;
}
}
void sortItems(struct Item items[], int n) {
qsort(items, n, sizeof(struct Item), compare);
}
```
3. 然后,实现贪心算法来选择物品放入背包。从排序后的物品数组中依次选择单位价值最高的物品放入背包,直到背包装满或者所有物品都被选择。例如:
```c
void knapsack(struct Item items[], int n, int capacity) {
sortItems(items, n);
int totalValue = 0;
int currentWeight = 0;
for (int i = 0; i < n; i++) {
if (currentWeight + items[i].weight <= capacity) {
currentWeight += items[i].weight;
totalValue += items[i].value;
}
}
printf("背包中物品的总价值为:%d\n", totalValue);
}
```
这样,你就可以调用`knapsack`函数来解决01背包问题了。注意,以上代码只是一个简单的示例,实际应用中可能需要根据具体情况进行适当的修改和优化。
阅读全文
相关推荐
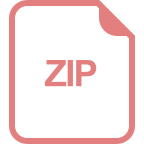
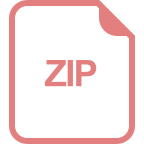
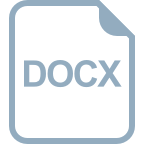















