python散点图拟合
时间: 2024-07-04 18:01:23 浏览: 359
在Python中,我们可以使用matplotlib和scipy库来创建散点图并进行拟合。Scatter plot(散点图)通常用来展示两个变量之间的关系,而拟合则是找到一条数学曲线或函数,使数据点尽可能接近这条曲线。
以下是创建散点图并进行简单线性拟合的基本步骤:
1. 导入所需库:
```python
import matplotlib.pyplot as plt
from scipy.optimize import curve_fit
import numpy as np
```
2. 准备数据(这里假设有一个x和y列表):
```python
x = np.array([...]) # x坐标数据
y = np.array([...]) # y坐标数据
```
3. 创建散点图:
```python
plt.scatter(x, y)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Scatter Plot with Linear Fit')
plt.grid(True)
plt.show()
```
4. 定义线性模型函数(这里假设是y = ax + b):
```python
def linear_model(x, a, b):
return a * x + b
```
5. 使用`curve_fit`函数进行拟合:
```python
params, _ = curve_fit(linear_model, x, y)
a_fit, b_fit = params
```
6. 绘制拟合的直线:
```python
x_fit = np.linspace(min(x), max(x), 100) # 创建拟合线的x值范围
y_fit = linear_model(x_fit, a_fit, b_fit)
plt.plot(x_fit, y_fit, 'r-', label='Fitted Line (a={:.2f}, b={:.2f})'.format(a_fit, b_fit))
```
7. 添加拟合线到散点图:
```python
plt.legend()
plt.show()
```
阅读全文
相关推荐
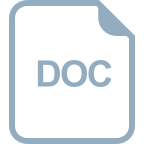
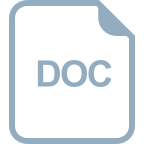
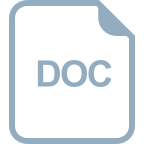















