编写一个学生和教师数据输入和显示程序,学生数据有编号、姓名、班号和成绩,教师数据有编号、姓名、职称和部门。要求将编号、姓名输入和显示设计成一个类person,并作为学生数据操作类student和教师数
时间: 2024-11-12 19:18:13 浏览: 7
为了编写一个学生和教师数据输入和显示的程序,我们可以首先创建一个名为`Person`的通用类,用于存储`编号`、`姓名`等基本信息。然后,基于这个类分别创建`Student`和`Teacher`两个子类,每个子类会添加特定的数据字段如`班号`、`成绩` 和 `职称`、`部门`。这里是一个简单的示例:
```python
class Person:
def __init__(self, id, name):
self.id = id
self.name = name
def input_data(self):
print("请输入{}的信息:".format(self.__class__.__name__))
self.id = int(input("编号: "))
self.name = input("姓名: ")
def display_data(self):
print("\n{}信息:\nID: {}\n姓名: {}".format(self.__class__.__name__, self.id, self.name))
class Student(Person):
def __init__(self, id, name, class_number, score):
super().__init__(id, name)
self.class_number = class_number
self.score = score
def input_data(self):
super().input_data()
self.class_number = int(input("班号: "))
self.score = float(input("成绩: "))
def display_data(self):
super().display_data()
print("班号: {}\n成绩: {}".format(self.class_number, self.score))
class Teacher(Person):
def __init__(self, id, name, title, department):
super().__init__(id, name)
self.title = title
self.department = department
def input_data(self):
super().input_data()
self.title = input("职称: ")
self.department = input("部门: ")
def display_data(self):
super().display_data()
print("职称: {}\n部门: {}".format(self.title, self.department))
# 使用示例
student = Student(0, "张三", 1, 85)
teacher = Teacher(1, "李四", "教授", "计算机科学系")
student.input_data()
student.display_data()
teacher.input_data()
teacher.display_data()
```
在这个例子中,`Person` 类负责基本的输入和显示功能,而`Student`和`Teacher`类则扩展了这些功能并添加了各自的特有属性。
阅读全文
相关推荐
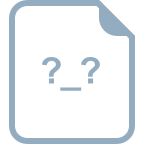
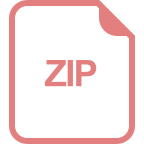











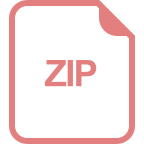