用c++编写一个学生和教师数据输入和显示程序,学生数据有编号、姓名、班号和成绩,教师数据有编号、姓名、职称和部门,要求将编号、姓名输入和显示设计成一个类person,并作为学生数据操作类student和教师数据操作类teacher的基类。
时间: 2024-02-05 18:02:28 浏览: 88
好的,我可以帮你解答这个问题。
首先,我们需要定义一个 `person` 类,包含编号和姓名两个成员变量,然后再定义 `student` 和 `teacher` 类,继承自 `person` 类,并添加各自的成员变量和方法。
以下是一份可能的实现代码:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class person {
public:
int id;
string name;
person(int id, string name) {
this->id = id;
this->name = name;
}
};
class student : public person {
public:
int class_no;
int score;
student(int id, string name, int class_no, int score) : person(id, name) {
this->class_no = class_no;
this->score = score;
}
void display() {
cout << "id: " << id << ", name: " << name << ", class_no: " << class_no << ", score: " << score << endl;
}
};
class teacher : public person {
public:
string title;
string department;
teacher(int id, string name, string title, string department) : person(id, name) {
this->title = title;
this->department = department;
}
void display() {
cout << "id: " << id << ", name: " << name << ", title: " << title << ", department: " << department << endl;
}
};
int main() {
vector<student> students;
students.push_back(student(1, "Tom", 1, 90));
students.push_back(student(2, "Jerry", 2, 80));
students.push_back(student(3, "Alice", 1, 95));
students.push_back(student(4, "Bob", 2, 85));
vector<teacher> teachers;
teachers.push_back(teacher(1, "John", "Professor", "Computer Science"));
teachers.push_back(teacher(2, "Mary", "Associate Professor", "Mathematics"));
cout << "Students:" << endl;
for (int i = 0; i < students.size(); i++) {
students[i].display();
}
cout << "Teachers:" << endl;
for (int i = 0; i < teachers.size(); i++) {
teachers[i].display();
}
return 0;
}
```
在上面的代码中,我们定义了 `person` 类作为基类,包含编号和姓名两个成员变量。然后,我们定义了 `student` 和 `teacher` 类,分别继承自 `person` 类,并添加各自的成员变量和方法。最后,在主函数中创建了几个学生和教师对象,并调用了它们的 `display()` 方法来显示数据。
希望这可以帮助你解决问题。
阅读全文
相关推荐
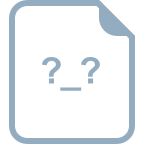
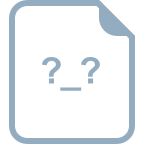













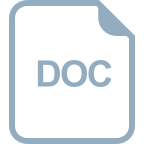