用c++ 编写一个学生和教师数据输入和显示程序,学生数据有编号、姓名、班号和成绩,教师数据有编号、姓名、职称和部门,要求将编号、姓名输入和显示设计成一个类person,并作为学生数据操作类student和教师数据操作类teacher的基类。
时间: 2024-03-14 22:48:50 浏览: 368
好的,下面是代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
class Person {
protected:
int id;
string name;
public:
Person(int id = 0, string name = ""): id(id), name(name) {}
void input() {
cout << "请输入编号:";
cin >> id;
cout << "请输入姓名:";
cin >> name;
}
void display() {
cout << "编号:" << id << " 姓名:" << name << endl;
}
};
class Student : public Person {
private:
int classNo;
double score;
public:
Student(int id = 0, string name = "", int classNo = 0, double score = 0): Person(id, name), classNo(classNo), score(score) {}
void input() {
Person::input();
cout << "请输入班号:";
cin >> classNo;
cout << "请输入成绩:";
cin >> score;
}
void display() {
Person::display();
cout << "班号:" << classNo << " 成绩:" << score << endl;
}
};
class Teacher : public Person {
private:
string title;
string department;
public:
Teacher(int id = 0, string name = "", string title = "", string department = ""): Person(id, name), title(title), department(department) {}
void input() {
Person::input();
cout << "请输入职称:";
cin >> title;
cout << "请输入部门:";
cin >> department;
}
void display() {
Person::display();
cout << "职称:" << title << " 部门:" << department << endl;
}
};
int main() {
Student stu;
Teacher tea;
cout << "请输入学生信息:" << endl;
stu.input();
cout << endl << "请输入教师信息:" << endl;
tea.input();
cout << endl << "学生信息:" << endl;
stu.display();
cout << endl << "教师信息:" << endl;
tea.display();
return 0;
}
```
上述代码中,我们定义了一个`Person`类作为学生、教师数据操作类的基类,该类包含编号和姓名两个成员变量以及输入和输出这两个方法。然后,我们分别定义了`Student`类和`Teacher`类,这两个类均继承了`Person`类,并增加了自己的成员变量和输入输出方法。最后在主函数中,我们分别创建`Student`类和`Teacher`类的对象,调用它们的输入输出方法即可实现学生和教师数据的输入和显示。
注意,我们在`input`和`display`方法中,使用了`Person::`来调用基类的同名方法,避免了子类和基类方法同名带来的歧义。
阅读全文
相关推荐
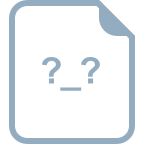













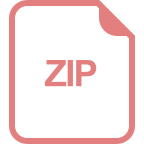